python九宫算术
时间: 2023-12-07 16:40:05 浏览: 147
以下是使用Python实现九宫算术的代码:
```python
import numpy as np
n = int(input("请输入矩阵的阶数:"))
a = np.zeros((n, n))
r, c = n // 2, n - 1 # 行,列
for i in range(n * n, 0, -1):
a[r][c] = i # 先填最大的数
r = (r + 1) % n # 取模(防止超出范围)
c = (c + 1) % n
if a[r][c] != 0: # 填数重复
r = (r - 1) % n # 退回原位
c = (c - 2) % n # 执行列-1
print(a)
```
这段代码使用了numpy库中的`np.zeros((n,n))`方法创建了一个n*n阶的矩阵,然后按照九宫算术的规则填充了矩阵,并输出了结果。
--相关问题--:
1. 九宫算术的规则是什么?
2. 如何使用Python实现数独游戏?
3. Python中如何生成随机数?
相关问题
用Python九宫算数
九宫算数是一种数学游戏,要求在一个3x3的九宫格中填入1-9的数字,使得每行、每列、对角线上的数字之和都相等。使用Python实现九宫算数可以通过以下步骤实现:
1. 导入itertools模块中的product()方法,使用该方法生成所有可能的数字组合。
2. 遍历所有组合,找出所有三个数和为15的组合。
3. 在上一步的基础上,找出使每行、每列、对角线和相等的所有组合。
4. 依次进行第一列求和,第二列求和、第三列求和、对角线求和、对角线求和。
5. 最后打印出九宫格。
以下是一个简单的Python代码实现九宫算数的例子:
```
import itertools
# 生成所有可能的数字组合
numbers = range(1, 10)
combinations = itertools.permutations(numbers)
# 遍历所有组合,找出所有三个数和为15的组合
sum_15_combinations = []
for combination in combinations:
if combination[0] + combination[1] + combination[2] == 15 and \
combination[3] + combination[4] + combination[5] == 15 and \
combination[6] + combination[7] + combination[8] == 15 and \
combination[0] + combination[3] + combination[6] == 15 and \
combination[1] + combination[4] + combination[7] == 15 and \
combination[2] + combination[5] + combination[8] == 15 and \
combination[0] + combination[4] + combination[8] == 15 and \
combination[2] + combination[4] + combination[6] == 15:
sum_15_combinations.append(combination)
# 打印所有符合条件的组合
for combination in sum_15_combinations:
print(combination[0], combination[1], combination[2])
print(combination[3], combination[4], combination[5])
print(combination[6], combination[7], combination[8])
print()
```
阅读全文
相关推荐
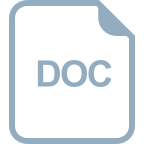
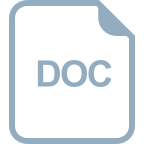
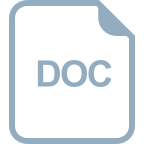
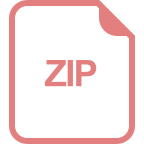
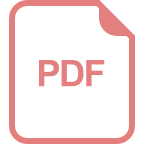
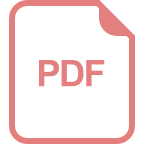
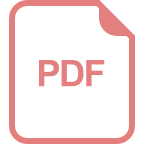
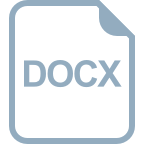
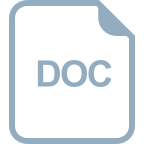
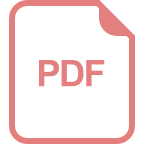
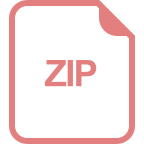
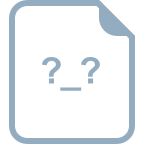
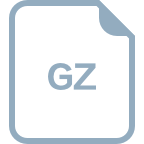
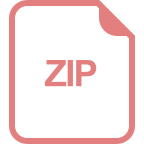
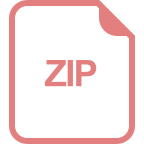