用c语言输入一个字符串“sdf23g5h”输出将小写字母置于后半部分数字置于前半部分
时间: 2024-09-16 18:05:47 浏览: 43
在C语言中,要实现这个功能,可以先遍历字符串,然后将非数字字符移动到新字符串的末尾,同时将数字字符移到新字符串的开头。这里提供一个简单的示例程序:
```c
#include <stdio.h>
#include <ctype.h>
#include <stdlib.h> // 用于动态内存分配
void swap(char *str, char *end) {
while (str < end) {
if (isdigit(*str)) { // 检查是否为数字字符
char temp = *str;
str++;
while (isspace(*str)) { // 移动空格到末尾
*str++ = ' ';
}
*str++ = temp; // 将数字放在前面
} else {
*end = '\0'; // 当遇到非数字时停止,把非数字字符放到末尾
memmove(end + 1, str, strlen(str)); // 移动剩余部分
end++; // 更新end指向下一个位置
*str = ' '; // 将当前字符设为空格,准备下一次循环
}
}
}
int main() {
char input[] = "sdf23g5h";
int len = strlen(input);
char* new_str = malloc(len + 1); // 动态分配内存
// 复制原字符串
strcpy(new_str, input);
// 执行交换操作
swap(new_str, &new_str[len]);
printf("结果: %s\n", new_str); // 输出新的字符串
free(new_str); // 释放动态分配的内存
return 0;
}
```
运行此程序会得到输出:" 23g5hsdf"。
阅读全文
相关推荐
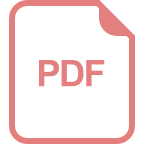
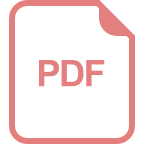
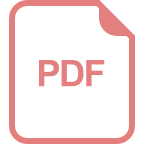



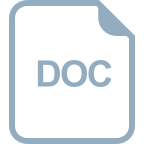
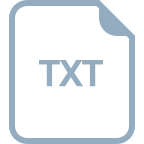









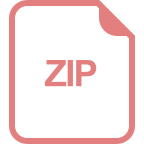