写一个unity c#脚本,实现屏幕中央准星放到物体上时显示一个panel,其显示物体的各种信息,准星离开后关闭panel
时间: 2024-09-26 12:18:54 浏览: 17
在Unity中,你可以通过C#编写如下的Mecanim或Input Manager脚本来实现这个功能。这里假设我们已经有了一个UI面板(GameObject)`uiPanel`,并且物体的信息已经封装在一个`ObjectInfo`类中:
```csharp
using UnityEngine;
using UnityEngine.UI;
public class AimAndPanel : MonoBehaviour
{
// UI Panel reference
public GameObject uiPanel;
// ObjectInfo class (replace with your actual implementation)
private ObjectInfo objectInfo;
// Camera reference for screen center aiming
private Camera mainCamera;
void Start()
{
mainCamera = Camera.main;
objectInfo = GetComponent<ObjectInfo>(); // Assuming the info is attached to this script
}
void Update()
{
// Check if cursor or aim point is near the center of the screen
Vector3 mousePosition = Input.mousePosition;
float mouseXToScreenCenterX = (mainCamera.ViewportToWorldPoint(mousePosition).x - mainCamera.ViewportToWorldPoint(new Vector3(0.5f, 0.5f)).x);
float mouseXToScreenCenterY = (mainCamera.ViewportToWorldPoint(mousePosition).y - mainCamera.ViewportToWorldPoint(new Vector3(0.5f, 0.5f)).y);
// Use a tolerance value (e.g., 5 pixels) for proximity check
const float tolerance = 5f;
if (Mathf.Abs(mouseXToScreenCenterX) < tolerance && Mathf.Abs(mouseXToScreenCenterY) < tolerance)
{
ShowPanel(objectInfo); // Display panel with object info
}
else
{
HidePanel(); // Close the panel when not aimed at center
}
}
private void ShowPanel(ObjectInfo objInfo)
{
uiPanel.SetActive(true);
// Set the UI panel's content with objectInfo data
Text text = uiPanel.GetComponent<Text>();
text.text = "Object Info:\n" + objInfo.ToString();
}
private void HidePanel()
{
uiPanel.SetActive(false);
}
}
// Example usage: Place an ObjectInfo component on the game object you want to display info about
[Serializable]
public class ObjectInfo
{
// Add properties here like name, position, etc.
public string Name { get; set; }
public Vector3 Position { get; set; }
// Override ToString() method for clean output in the UI
public override string ToString()
{
return $"Name: {Name}, Position: ({Position.x}, {Position.y})";
}
}
```
相关推荐
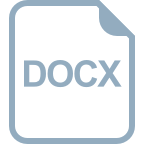
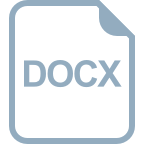
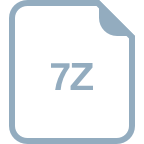















