sectionList的分组导航条
时间: 2024-09-18 13:18:46 浏览: 31
SectionList在React Native中是一种用于展示分组内容的组件,它通常被用于构建类似新闻聚合、产品目录或详细列表的应用场景中。它允许将数据按照一定的分类(section)进行组织,并显示成一系列有标题的小组件。每个section可以包含多个项(items),用户可以通过滚动浏览不同的组别。
SectionList的主要属性包括`renderSectionHeader`来提供每个section的头部视图,` renderItem`则用于渲染每一个list item的内容。通过`data`属性传递给组件的数据数组应该是一个嵌套的对象结构,比如:
```javascript
[
{
title: 'Section 1',
data: [
{ key: 'item1', ... },
{ key: 'item2', ... }
]
},
{
title: 'Section 2',
data: [...]
}
]
```
用户交互如点击section头或item时,可以通过事件处理函数来响应相应的操作。SectionList提供了良好的性能优化,当滚动到一个新的section或item时才加载其对应的数据,提高了用户体验。
相关问题
如何根据数据做sectionList的首字母导航条,点击字母可以跳转到对应的section
在React Native中创建一个根据数据做首字母导航条(通常称为A-Z导航)并配合SectionList的实现,你需要经历以下几个步骤:
1. **获取首字母**:首先,遍历SectionList的数据,对每个section的title取首字母,存储在一个对象(例如`alphabetIndexMap`)中,键为字母,值为包含该字母section的数组。
```javascript
const alphabetIndexMap = {};
Object.entries(data).forEach(([index, { title }]) => {
const firstLetter = title.charAt(0);
if (!alphabetIndexMap[firstLetter]) {
alphabetIndexMap[firstLetter] = [index];
} else {
alphabetIndexMap[firstLetter].push(index);
}
});
```
2. **渲染导航栏**:创建一个头部组件(如`AlphabetNavigator`),显示字母列表,每个字母链接到一个跳转函数,传入对应的数据索引数组。
```javascript
function AlphabetNavigator({ onPress }) {
return (
<View>
{Object.keys(alphabetIndexMap).map((letter) => (
<TouchableOpacity
key={letter}
onPress={() => onPress(letter)}
>
<Text>{letter}</Text>
</TouchableOpacity>
))}
</View>
);
}
```
3. **处理点击事件**:在SectionList的顶部组件中,当你点击字母导航时,调用`onPress`函数,然后根据传入的字母从`alphabetIndexMap`中获取相应section的索引数组,跳转到SectionList的相应位置。
```javascript
<SectionList
sections={data}
headerComponent={<AlphabetNavigator onPress={(letter) => handleNavigation(letter)} />}
// ...
/>
```
4. **跳转到section**:在`handleNavigation`函数内部,你可以使用`scrollToSection`方法滚动到指定section。
```javascript
function handleNavigation(letter) {
const targetSections = alphabetIndexMap[letter];
SectionList.scrollToSection({ index: targetSections[0], animated: true });
}
```
我说的是右侧的垂直的导航条,而且为什么点击了但是没有跳转到精确的位置,每个section的数据数量不同
对于右侧垂直导航条的问题,你需要确保它连接的是SectionList的正确索引,并考虑到每个section的数据长度差异。以下是可能出现问题及解决方法:
1. **确保索引准确**:检查你在生成导航条时是否按照数据标题的第一个字母计算了正确的索引。由于section数据量不同,你可能需要使用section的起始位置而不是数据总数作为索引。
2. **动态高度调整**:SectionList的高度可能会因为内容长度变化而有所不同。使用`stickyHeaderIndices`属性,结合`FlatList`的`extraData`特性,可以确保每个section始终处于可见状态。
```jsx
//...
<ListView
stickyHeaderIndices={['yourFirstSectionKey', 'yourSecondSectionKey']}
extraData={this.state}
//...
</ListView>
```
3. **滚动到正确位置**:当用户点击字母后,计算出目标section的准确位置(可能是起始位置或者某个特定元素位置)。然后,调用`scrollToRowAtIndexPath`方法,将焦点移动到该位置。
```jsx
handleNavigation(letter) {
const targetSection = data.find(item => item.title[0] === letter);
const sectionIndex = targetSection.key;
const itemIndex = targetSection.data.length > 0 ? 0 : null; // 根据数据量决定是否直接滚动到底部
this.listView.scrollToRowAtIndexPath({rowId: sectionIndex, atEnd: false}, {animated: true});
}
```
如果仍然无法正确跳转,还需要排查代码中其他可能导致定位错误的地方,比如更新状态或调用滚动方法的部分是否有误。
阅读全文
相关推荐
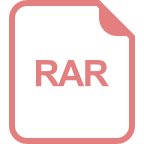
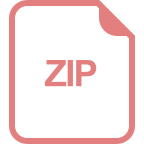
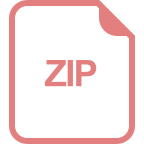
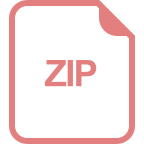



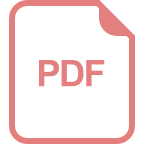
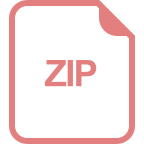
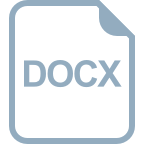
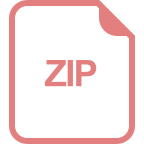
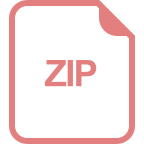