uniapp中input只能输入数字
时间: 2023-11-14 10:09:47 浏览: 454
可以通过在input标签中添加type="number"属性来限制只能输入数字,同时也可以通过监听input事件,在输入内容发生变化时进行正则匹配,只保留数字部分。
示例代码:
```html
template>
<div>
<input type="number" v-model="num">
</div>
</template>
<script>
export default {
data() {
return {
num: ''
}
},
watch: {
num(val) {
// 只保留数字部分
this.num = val.replace(/[^\d]/g, '')
}
}
}
</script>
```
相关问题
uniapp input框只能输入数字
您可以使用uni-app的input组件的type属性将输入框设置为数字类型,这样用户只能输入数字。
示例代码:
```
<template>
<view>
<input type="number" v-model="inputValue" placeholder="请输入数字" />
</view>
</template>
<script>
export default {
data() {
return {
inputValue: ''
}
}
}
</script>
```
在上面的示例中,我们将input组件的type属性设置为number,这样用户只能输入数字。在data中定义了一个inputValue变量,用于保存用户输入的内容。使用v-model指令将input组件与inputValue变量进行双向绑定,这样当用户输入数字时,inputValue变量也会相应地更新。
uniapp input只能输入数字
### 回答1:
uniapp的input组件默认是可以输入任何字符的,如果需要限制只能输入数字,可以通过以下两种方式实现:
1. 使用type属性设置为number,这样输入框就只能输入数字了。
```html
<uni-input type="number"></uni-input>
```
2. 在input事件中判断输入的字符是否为数字,如果不是则阻止输入。
```html
<uni-input @input="handleInput"></uni-input>
```
```javascript
methods: {
handleInput(event) {
const value = event.detail.value
if (!/^\d*$/.test(value)) {
event.detail.value = value.replace(/[^\d]/g, '')
}
}
}
```
以上两种方式都可以实现只能输入数字的效果,具体使用哪种方式取决于实际需求。
### 回答2:
Uniapp是一种基于Vue框架开发的跨平台应用程序框架,支持多种移动端和Web端平台。它提供了一系列的组件和API来帮助我们轻松地构建跨平台应用。在Uniapp中,对于input控件只能输入数字这一问题,可以通过以下的方法解决。
首先,在Uniapp中,我们可以使用input控件的type属性来限制输入内容的类型。其中,type="number"即表示只能输入数字。这种方式可以在大部分情况下解决只输入数字的问题:
```
<template>
<input type="number" v-model="inputValue">
</template>
```
这里的v-model指令用于实现数据的双向绑定,对应的是在data中定义的inputValue变量。这样,用户在输入input控件中的内容时,只能输入数字,如果输入其他字符会被自动过滤。
但是,这种方式同时也存在一些问题。比如,当输入框中只有一个小数点时,就无法符合我们的输入要求了。又或者,当输入框中还需要输入其他特殊字符时,也无法满足需求。因此,需要进一步的处理。
一种解决方式是通过正则表达式来限制输入框中的内容。通过在输入框的onInput事件中对输入字符进行正则匹配,可以只允许数字和特定字符的输入,其他字符都会被忽略:
```
<template>
<input @input="onInput" v-model="inputValue">
</template>
<script>
export default {
data() {
return {
inputValue: ''
}
},
methods: {
onInput(e) {
// 只允许数字和小数点
e.target.value = e.target.value.replace(/[^\d.]/g, '')
// 过滤多余的小数点
e.target.value = e.target.value.replace(/\.{2,}/g, '.')
// 只允许一个小数点
e.target.value = e.target.value.replace('.', '$#$').replace(/\./g, '').replace('$#$', '.')
// 可以自定义添加其他字符的正则表达式
}
}
}
</script>
```
这里的onInput方法中,通过正则表达式对输入的字符进行过滤和替换,只允许数字和小数点,最终通过v-model绑定到变量inputValue上。
当需要过滤特定字符时,只需要在正则表达式中添加对应的字符即可。
总之,在Uniapp中,通过type属性和正则表达式,我们可以轻松地实现input控件只能输入数字的功能,同时也可以根据实际需求进行进一步的扩展和优化。
### 回答3:
uniapp是一种跨平台开发框架,它可以用来开发多个平台上的应用程序。在uniapp中,input组件是常用的用户输入组件,可以用来接收用户输入的文字或数字等。但是有时候我们需要限制用户只能输入数字,那么该如何实现呢?
首先,我们可以使用uniapp中的input组件的type属性来控制输入框的类型,将其设置为number类型,这样就只能输入数字了,代码如下:
```
<template>
<view class="content">
<input type="number" v-model="inputValue" placeholder="请输入数字" />
<button @click="submit">提交</button>
</view>
</template>
<script>
export default {
data() {
return {
inputValue: ""
};
},
methods: {
submit() {
console.log("提交的数字为:", this.inputValue);
}
}
};
</script>
```
上述代码中,我们将input的类型设置为number,这样就限制了用户只能输入数字,同时我们还设置了一个placeholder属性,提示用户输入内容的类型。然后我们在submit方法中,打印出用户输入的数字。
此外,我们也可以使用正则表达式来限制输入的内容必须为数字,当用户输入其他字符时自动过滤掉。具体实现代码如下:
```
<template>
<view class="content">
<input type="text" v-model="inputValue" @input="filterValue" placeholder="请输入数字" />
<button @click="submit">提交</button>
</view>
</template>
<script>
export default {
data() {
return {
inputValue: ""
};
},
methods: {
filterValue() {
// 使用正则表达式过滤输入内容
this.inputValue = this.inputValue.replace(/[^\d]/g, "");
},
submit() {
console.log("提交的数字为:", this.inputValue);
}
}
};
</script>
```
上述代码中,我们先将input的类型设置为text,不再限制用户只能输入数字,而是在input事件中,使用正则表达式过滤输入内容,只保留数字,然后将过滤后的数字赋值给inputValue。这样,当用户输入的不是数字时,就会自动过滤掉。
综上所述,uniapp中input只能输入数字的实现方法有两种,一种是将input类型设置为number,另一种是使用正则表达式过滤输入内容。具体选择哪一种方法,可以根据实际需求和场景来决定。
阅读全文
相关推荐
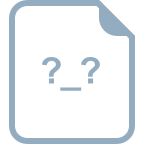













