Arrays.sort();
时间: 2024-06-14 14:08:18 浏览: 14
Arrays.sort()是Java中用于对数组进行排序的方法。它使用了快速排序算法来对数组元素进行排序。快速排序是一种高效的排序算法,它通过将数组分成较小的子数组,并对这些子数组进行排序,最终将它们合并起来得到有序的数组。
使用Arrays.sort()方法可以对包含基本数据类型或对象的数组进行排序。对于基本数据类型的数组,它会按照元素的大小进行升序排序。对于对象数组,它会根据对象的比较规则进行排序,通常是通过对象的compareTo()方法或Comparator接口来实现。
以下是Arrays.sort()方法的一些重要特点:
1. 对于基本数据类型的数组,它使用了双轴快速排序算法,具有较高的性能。
2. 对于对象数组,它要求对象实现Comparable接口或者通过Comparator接口提供比较规则。
3. 可以通过传递Comparator对象作为参数来自定义排序规则。
4. 排序是原地进行的,即不会创建新的数组,而是直接在原始数组上进行排序。
使用Arrays.sort()方法非常简单,只需将待排序的数组作为参数传递给该方法即可。以下是一个示例代码:
```
int[] arr = {5, 2, 8, 1, 9};
Arrays.sort(arr);
for (int num : arr) {
System.out.print(num + " ");
}
```
输出结果为:1 2 5 8 9,表示数组已经按照升序排序。
相关问题
arrays.sort
`Arrays.sort()` 是 Java 中用于对数组进行排序的方法。它可以对任何类型的数组进行排序,包括基本类型和对象类型。排序时可以使用默认的排序顺序,也可以自定义排序顺序。默认情况下,它使用快速排序算法进行排序。
下面是一个使用 `Arrays.sort()` 方法对整数数组进行排序的示例:
```java
int[] arr = {5, 3, 8, 2, 7};
Arrays.sort(arr);
System.out.println(Arrays.toString(arr)); // [2, 3, 5, 7, 8]
```
在上面的示例中,我们首先创建了一个整数数组 `arr`,然后使用 `Arrays.sort()` 方法对其进行排序。最后,我们使用 `Arrays.toString()` 方法将排序后的数组转换为字符串并打印出来。
需要注意的是,`Arrays.sort()` 方法会改变原始数组的顺序,如果不想改变原始数组的顺序,可以先将原始数组复制一份再进行排序。
Arrays.sort
arrays.sort和collection.sort都是用于对数组或集合进行排序的方法。
arrays.sort是Java中Arrays类提供的静态方法,可以对数组进行排序。它的使用方法是:Arrays.sort(数组名)。其中,数组名是需要排序的数组的名称。
collection.sort是Java中Collections类提供的静态方法,可以对集合进行排序。它的使用方法是:Collections.sort(集合名)。其中,集合名是需要排序的集合的名称。
两者的区别在于,arrays.sort只能对数组进行排序,而collection.sort可以对各种类型的集合进行排序,包括List、Set等。此外,collection.sort还可以通过传入一个Comparator对象来实现自定义排序规则。
相关推荐
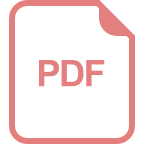
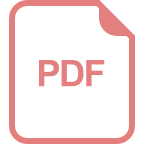
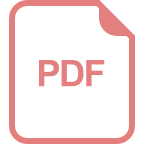












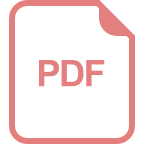
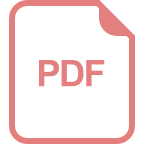