Java编写一个 Book 类,用于描述个人藏书,包含作者 Author、书名 Tile、价格 Price、出版社 Publisher、出版年份 Published Year共5个属性。然后编写一个测
时间: 2024-10-23 15:04:02 浏览: 70
好的,我可以帮你创建一个简单的 `Book` 类,并提供一个测试示例。首先,我们定义 `Author` 和 `Book` 类:
```java
// 定义 Author 类
public class Author {
private String name;
public Author(String name) {
this.name = name;
}
// 获取和设置名字的方法
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
// 定义 Book 类
public class Book {
private Author author;
private String title;
private double price;
private String publisher;
private int publishedYear;
// 构造函数接受所有属性的值
public Book(Author author, String title, double price, String publisher, int publishedYear) {
this.author = author;
this.title = title;
this.price = price;
this.publisher = publisher;
this.publishedYear = publishedYear;
}
// 获取和设置各个属性的方法
public Author getAuthor() {
return author;
}
public void setAuthor(Author author) {
this.author = author;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public String getPublisher() {
return publisher;
}
public void setPublisher(String publisher) {
this.publisher = publisher;
}
public int getPublishedYear() {
return publishedYear;
}
public void setPublishedYear(int publishedYear) {
this.publishedYear = publishedYear;
}
}
```
接下来,我们可以编写一个简单的测试类来演示如何创建和操作 `Book` 对象:
```java
public class Main {
public static void main(String[] args) {
// 创建 Author 对象
Author author = new Author("J.K. Rowling");
// 创建 Book 对象
Book book = new Book(author, "Harry Potter and the Philosopher's Stone", 29.99, "Bloomsbury Publishing", 1997);
// 输出 Book 的信息
System.out.println("Author: " + book.getAuthor().getName());
System.out.println("Title: " + book.getTitle());
System.out.println("Price: " + book.getPrice());
System.out.println("Publisher: " + book.getPublisher());
System.out.println("Published Year: " + book.getPublishedYear());
// 修改 Book 属性并再次输出
book.setTitle("Harry Potter and the Chamber of Secrets");
book.setPrice(34.99);
book.setPublishedYear(1998);
System.out.println("\nUpdated:\n" + "Title: " + book.getTitle());
System.out.println("Price: " + book.getPrice());
System.out.println("Published Year: " + book.getPublishedYear());
}
}
```
在这个例子中,我们展示了如何创建 `Book` 对象,以及如何通过 `Book` 类的 getter 和 setter 方法访问和修改其属性。
阅读全文
相关推荐
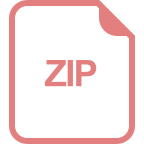
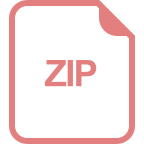
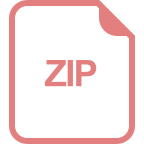
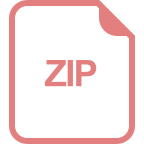
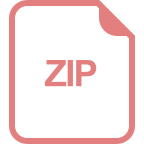
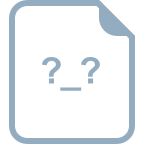
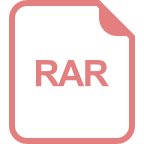
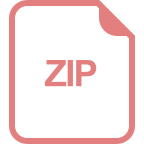
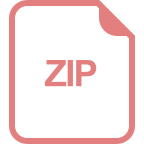
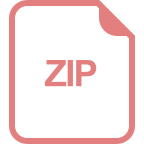
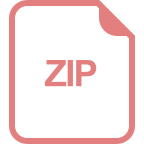
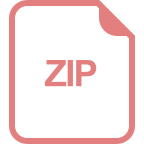
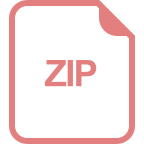
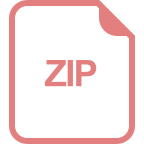
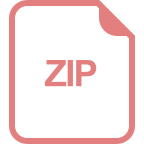
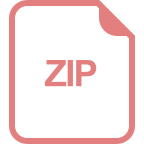
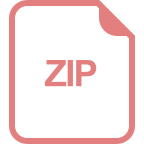
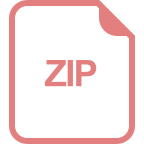

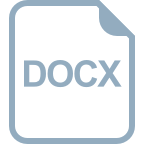