用uvm语言写一个对fifo rtl的验证环境,包括 trans sequence driver monitor 等
时间: 2024-09-13 14:17:17 浏览: 60
UVM(Universal Verification Methodology)是一种用于验证复杂数字设计的系统级方法学,它是IEEE标准的一部分(IEEE 1800.2)。UVM利用面向对象的编程原则构建了一个验证平台,其中包括各种组件,如序列器(sequencer)、驱动器(driver)、监视器(monitor)、代理(agent)、得分板(scoreboard)和环境(environment)。下面是一个简化的UVM验证环境的例子,用于验证FIFO(First-In-First-Out)RTL模块。
首先,我们需要定义一个transaction类,它代表FIFO验证中将要进行的事务。
```verilog
class fifo_transaction extends uvm_sequence_item;
rand bit [7:0] data; // 假设FIFO数据宽度为8位
rand bit wr_en; // 写使能信号
rand bit rd_en; // 读使能信号
// 其他属性...
// 构造函数
function new(string name = "fifo_transaction");
super.new(name);
endfunction
// 标准的UVM宏,用于各种事务的操作
`uvm_object_utils_begin(fifo_transaction)
`uvm_field_int(data, UVM_ALL_ON)
`uvm_field_int(wr_en, UVM_ALL_ON)
`uvm_field_int(rd_en, UVM_ALL_ON)
// 其他宏...
`uvm_object_utils_end
endclass
```
接下来,我们创建一个sequence类,它用于产生事务序列。
```verilog
class fifo_sequence extends uvm_sequence #(fifo_transaction);
// 构造函数
function new(string name = "fifo_sequence");
super.new(name);
endfunction
virtual task body();
fifo_transaction transaction;
repeat (10) begin
transaction = fifo_transaction::type_id::create(.name("transaction"), .contxt(get_full_name()));
start_item(transaction);
if (!transaction.randomize()) `uvm_error("RANDOMIZE", "Transaction randomization failed")
finish_item(transaction);
end
endtask
endclass
```
然后,创建一个driver类,它将从sequencer接收事务并驱动它们到DUT(Design Under Test)。
```verilog
class fifo_driver extends uvm_driver #(fifo_transaction);
virtual fifo_if fifo_vif; // 假设有一个FIFO接口
// 构造函数
function new(string name = "fifo_driver", uvm_component parent);
super.new(name, parent);
endfunction
virtual function void build_phase(uvm_phase phase);
if (!uvm_config_db#(virtual fifo_if)::get(this, "", "fifo_vif", fifo_vif)) begin
`uvm_error("NO_VIF", {"Virtual interface must be set for: ", get_full_name()})
end
endfunction
virtual task run_phase(uvm_phase phase);
forever begin
seq_item_port.get_next_item(req);
// 驱动信号到FIFO的DUT
// ...
seq_item_port.item_done();
end
endtask
endclass
```
监视器monitor用于监听总线事务,捕获FIFO接口上的活动,并将信息传递给scoreboard。
```verilog
class fifo_monitor extends uvm_monitor;
virtual fifo_if fifo_vif;
uvm_analysis_port #(fifo_transaction) mon_analysis_port;
// 构造函数
function new(string name = "fifo_monitor", uvm_component parent);
super.new(name, parent);
endfunction
virtual function void build_phase(uvm_phase phase);
mon_analysis_port = new(.name("mon_analysis_port"), .parent(this));
if (!uvm_config_db#(virtual fifo_if)::get(this, "", "fifo_vif", fifo_vif)) begin
`uvm_error("NO_VIF", {"Virtual interface must be set for: ", get_full_name()})
end
endfunction
virtual task run_phase(uvm_phase phase);
// 实时捕获FIFO的读写操作,并发送事务
// ...
endtask
endclass
```
最后,我们需要将这些组件集成到一个环境中,并添加scoreboard和agent。
```verilog
class fifo_env extends uvm_env;
// 定义components
fifo_driver driver;
fifo_monitor monitor;
fifo_scoreboard scoreboard;
fifo_agent agent;
// 其他组件...
// 构造函数
function new(string name = "fifo_env", uvm_component parent);
super.new(name, parent);
endfunction
// build_phase和connect_phase
// ...
// run_phase
// ...
endclass
```
请注意,为了构建一个完整的UVM验证环境,需要编写大量的代码,并且需要对UVM框架有深入的理解。上述代码段只是一个简单的概述,实际实现会更加复杂,需要考虑事务处理、时序控制、报告机制以及与DUT的连接等问题。
阅读全文
相关推荐
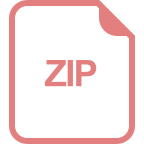
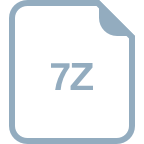



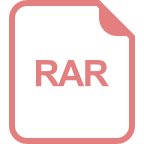
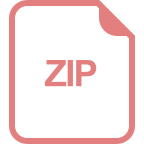
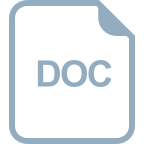
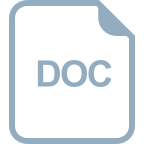








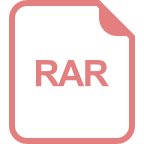