如何设计前端窗口呢?,要求实现连接mssms数据库(用Windows自带身份认证)并从中提取账号(employeeID)和密码(password)进行登录,登录之后进入员工信息界面显示员工各项信息
时间: 2024-11-25 19:19:44 浏览: 5
要设计一个前端窗口,用于连接到 MSSMS 数据库并实现 Windows 自带身份验证的登录功能,你需要遵循以下步骤:
1. **前端开发**:
使用 HTML、CSS 和 JavaScript 构建用户界面。你可以选择 React、Vue 或 Angular 这样的框架,它们提供了丰富的组件化开发能力。
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Employee Login</title>
<!-- 引入你的 CSS 文件 -->
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="login-form">
<form id="loginForm">
<input type="text" placeholder="Employee ID" id="employeeId" required>
<input type="password" placeholder="Password" id="password" required>
<button type="submit">Login</button>
<p class="error" id="errorMessage"></p>
</form>
</div>
<script src="scripts.js"></script>
</body>
</html>
```
1. **JavaScript 部分 (scripts.js)**:
- 使用 Fetch API 或 Axios 发送异步请求到服务器,处理登录逻辑。
- 使用内置的 Windows Identity Foundation (WIF) 或 Active Directory Authentication Library (ADAL) 来处理身份验证。
```javascript
document.getElementById('loginForm').addEventListener('submit', async function(e) {
e.preventDefault();
const employeeId = document.getElementById('employeeId').value;
const password = document.getElementById('password').value;
try {
// 假设这里有个函数,实际使用时需要替换为连接到 MSSMS 的代码
const response = await fetch('/api/login', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ employeeId, password }),
});
if (response.ok) {
// 登录成功后跳转到员工信息页面或隐藏错误消息
window.location.href = '/employee-profile';
} else {
document.getElementById('errorMessage').innerText = 'Invalid credentials.';
}
} catch (error) {
console.error(error);
document.getElementById('errorMessage').innerText = 'An error occurred.';
}
});
```
2. **后端服务(假设使用Node.js + Express)**:
- 创建一个 Express 应用,处理登录请求,检查数据库中的凭证是否匹配。
- 如果使用 Mssql 驱动,安装 `tedious` 或 `mssql` 包。
```javascript
const express = require('express');
const app = express();
const bodyParser = require('body-parser');
app.use(bodyParser.json());
// 假设数据库配置在这里
const config = { /* ... */ };
const pool = new mssql.Connection(config);
app.post('/api/login', async (req, res) => {
try {
const sqlQuery = `
SELECT * FROM Employees
WHERE EmployeeID = @employeeId AND Password = @password
AND windows_username = ${process.env.USERNAME}
`;
const result = await pool.request().query(sqlQuery, { employeeId: req.body.employeeId, password: req.body.password });
if (result.rowCount === 1) {
res.status(200).json({ success: true });
} else {
res.status(401).json({ error: 'Invalid credentials.' });
}
} catch (err) {
console.error(err);
res.status(500).json({ error: 'Database connection failed.' });
}
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
```
3. **安全性注意事项**:
- 不要在前端直接存储敏感数据如密码,而是在服务器上加密存储。
- 使用 HTTPS 提供安全的数据传输。
4. **权限管理**:
- 在数据库层面设置访问控制,确保只有授权用户才能查看员工信息。
完成以上步骤后,当用户填写正确的员工ID和密码并通过Windows身份验证,他们将能够登录并看到员工信息。如果你对某个环节有具体的问题,请随时提问。
阅读全文
相关推荐
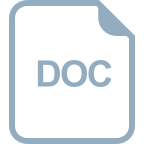
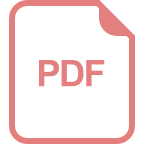



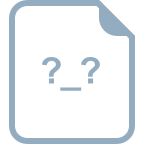
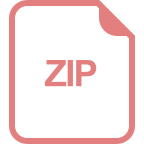
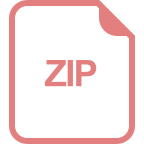
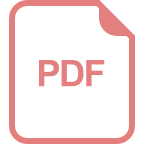
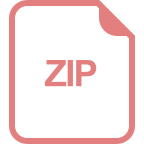
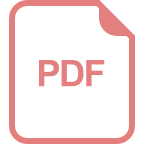
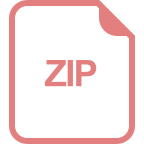
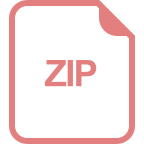
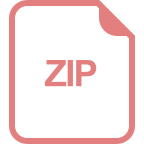
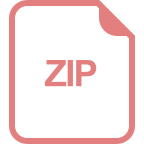
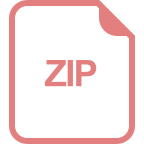