c语言字符串数组查找
时间: 2023-11-27 20:46:10 浏览: 284
在C语言中,可以使用以下函数来查找字符串数组中的元素:
1. strcmp()函数:用于比较两个字符串是否相等,如果相等则返回0,否则返回非0值。
2. strstr()函数:用于在一个字符串中查找另一个字符串,如果找到则返回第一次出现的位置,否则返回NULL。
3. strchr()函数:用于在一个字符串中查找指定字符,如果找到则返回第一次出现的位置,否则返回NULL。
4. strrchr()函数:用于在一个字符串中查找指定字符,如果找到则返回最后一次出现的位置,否则返回NULL。
以下是一个示例代码,演示如何使用strchr()函数在字符串数组中查找指定字符并打印结果:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[5][20] = {"apple", "banana", "orange", "grape", "pear"};
char c = 'a';
char *ppos;
for (int i = 0; i < 5; i++) {
ppos = strchr(str[i], c);
if (ppos != NULL) {
printf("Found '%c' in '%s' at position %ld\n", c, str[i], ppos - str[i]);
}
}
return 0;
}
```
输出结果为:
```
Found 'a' in 'apple' at position 0
Found 'a' in 'banana' at position 1
Found 'a' in 'grape' at position 3
```
阅读全文
相关推荐
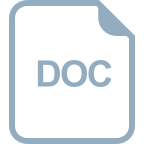
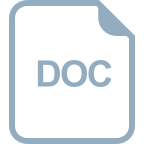
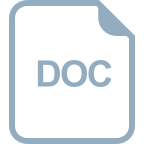
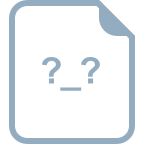
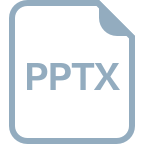
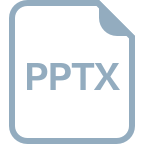
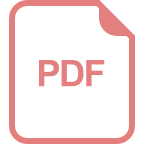
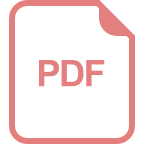
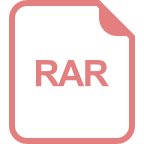
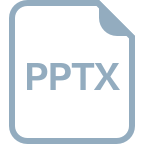
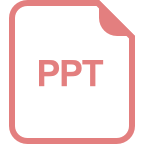
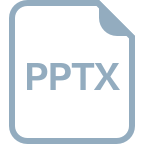
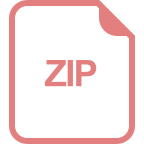
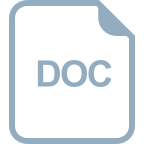
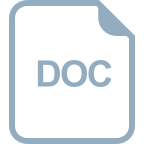
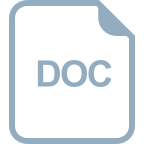
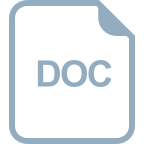
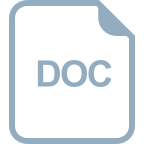