Status CreateList( ) 函数从键盘读入线性表中数据元素个数n,再依次读入n 个数据元素。若读入的n 是负值,则视为读入0;取n个数,用c语言创建单链表,将代码写出来
时间: 2024-09-26 21:13:11 浏览: 56
在C语言中,创建一个名为`Status CreateList()`的函数用于输入线性表的数据元素并构造单链表的过程可以大致按照以下步骤:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data; // 节点存储的数据
struct Node* next; // 指向下一个节点的指针
} Node;
// 创建新节点并分配内存
Node* createNode(int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (!newNode) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->data = value;
newNode->next = NULL;
return newNode;
}
// 功能函数 Status CreateList()
Status CreateList() {
int n, i;
// 询问用户输入数据元素个数,并处理异常情况
printf("Enter the number of elements or a negative value for 0: ");
scanf("%d", &n);
if (n < 0) {
n = 0;
} else if (n == 0 || n > INT_MAX / sizeof(Node)) {
printf("Input exceeds the limit, assuming 0.\n");
n = 0;
}
// 初始化链表头指针
Node* head = NULL;
// 遍历并添加元素到链表
for (i = 0; i < n; i++) {
int input;
printf("Enter element %d: ", i+1);
scanf("%d", &input);
// 创建新节点并将其添加到链表
Node* newNode = createNode(input);
if (head == NULL) {
head = newNode;
} else {
Node* temp = head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
}
// 如果链表为空,说明输入错误或选择0
if (head == NULL) {
printf("Failed to create list.\n");
return STATUS_FAIL;
} else {
printf("List created successfully.\n");
return STATUS_SUCCESS;
}
}
// 使用示例:
int main() {
Status status = CreateList();
return 0;
}
```
在这个代码中,`CreateList()`函数首先获取用户输入,然后创建一个单链表。如果输入有效,它会创建对应数量的节点并将它们连接起来。如果输入无效,例如输入的是负值或者超出限制,函数会相应地给出提示。
阅读全文
相关推荐







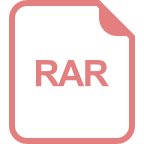







