用C语言编写“图像压缩问题”的动态规划算法代码
时间: 2024-10-18 22:07:07 浏览: 38
在C语言中,动态规划通常用于解决最优化问题,比如图像压缩可以通过选择最优的编码方式来降低数据量。这里简单展示一个基于哈夫曼编码的图像压缩算法示例,它是一种常见的无损压缩方法。请注意,这只是一个基本框架,实际应用中可能会更复杂:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义节点结构体
typedef struct {
char symbol;
int freq; // 符号出现频率
struct node* left, *right; // 左右子树指针
} Node;
// 动态规划辅助函数
int getMin(int a, int b) { return a < b ? a : b; }
// 创建新的哈夫曼树节点
Node* createNode(char symbol, int freq) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->symbol = symbol;
newNode->freq = freq;
newNode->left = newNode->right = NULL;
return newNode;
}
// 构建哈夫曼树并返回根节点
Node* buildHuffmanTree(int arr[], int n) {
// 先将频率数组转换为优先队列(最小堆)
priority_queue<Node*, vector<Node*>, compare> huffmanQueue(arr, n);
while (huffmanQueue.size() > 1) {
// 弹出两个频率最低的节点
Node* node1 = huffmanQueue.top();
huffmanQueue.pop();
Node* node2 = huffmanQueue.top();
huffmanQueue.pop();
// 新节点的频率为两节点之和,合并为新节点
int combinedFreq = node1->freq + node2->freq;
Node* newNode = createNode('\0', combinedFreq);
newNode->left = node1;
newNode->right = node2;
// 将新节点放回堆中
huffmanQueue.push(newNode);
}
return huffmanQueue.top(); // 返回剩余唯一的树顶节点
}
// 编码过程
void huffmanCoding(Node* root, char* compressedData, int* codeLength, char** compressedSymbols, int dataIndex, char symbol) {
if (root == NULL || root->symbol == symbol) {
// 如果达到叶子节点或找到当前符号,记录到压缩数据和长度数组
compressedSymbols[dataIndex] = root->symbol;
codeLength[symbol] = dataIndex++;
} else {
huffmanCoding(root->left, compressedData, codeLength, compressedSymbols, dataIndex, symbol);
huffmanCoding(root->right, compressedData, codeLength, compressedSymbols, dataIndex, symbol);
}
}
// 主函数 - 图像压缩
void imageCompression(char inputData[], int imageDataSize) {
// 省略实际图像分析获取字符频率部分,假设freqArr存储了每个像素值出现的次数
// 创建节点数组
Node* nodes = malloc(imageDataSize * sizeof(Node));
for (int i = 0; i < imageDataSize; ++i) {
nodes[i] = createNode(inputData[i], freqArr[i]);
}
// 构建哈夫曼树
Node* huffmannRoot = buildHuffmanTree(nodes, imageDataSize);
// 初始化压缩变量
char* compressedData = (char*)malloc(2 * imageDataSize); // 预计最大压缩比为2
int* codeLength = (int*)malloc(imageDataSize);
memset(codeLength, 0, imageDataSize * sizeof(int));
// 开始编码
huffmanCoding(huffmannRoot, compressedData, codeLength, NULL, 0, '\0');
// 输出压缩后的数据
printf("Compressed Data: %s\n", compressedData);
printf("Code Length: [");
for (int i = 0; i < imageDataSize; ++i) {
printf("%d ", codeLength[i]);
}
printf("]\n");
// 清理内存
free(compressedData);
free(codeLength);
for (int i = 0; i < imageDataSize; ++i) {
free(nodes[i]);
}
free(nodes);
}
// 示例输入数据
int main() {
char inputData[] = "YourImageDataHere"; // 替换成实际图像数据
int imageSize = sizeof(inputData);
imageCompression(inputData, imageSize);
return 0;
}
```
阅读全文
相关推荐
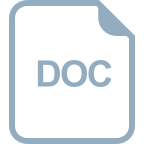
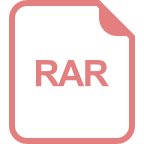
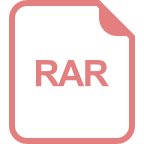

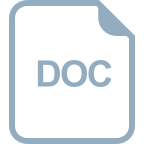
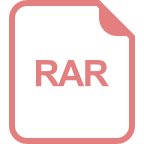
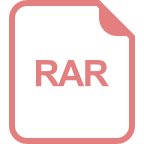
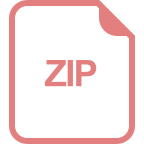
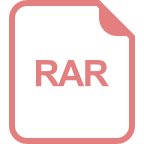
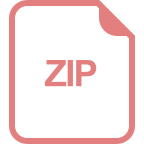
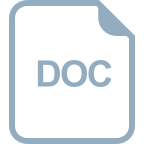
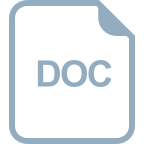
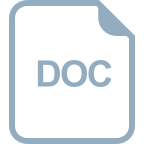
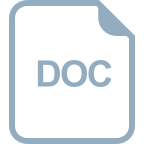
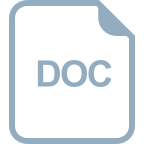
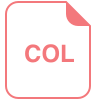
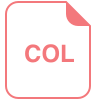
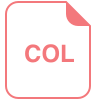
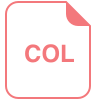