java 给二维码上添加LOGO字体
时间: 2023-12-08 15:04:59 浏览: 116
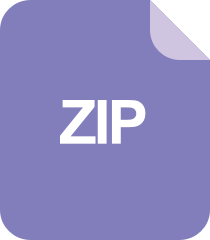
Java实现 生成 带 Logo 二维码
在Java中给二维码上添加字体Logo,你可以使用第三方库,如zxing和javase等。下面是一个使用zxing库的示例代码:
```java
import java.awt.Graphics2D;
import java.awt.image.BufferedImage;
import java.io.File;
import javax.imageio.ImageIO;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.EncodeHintType;
import com.google.zxing.MultiFormatWriter;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel;
public class QRCodeGenerator {
private static final int QRCODE_WIDTH = 300;
private static final int QRCODE_HEIGHT = 300;
private static final int LOGO_WIDTH = 80;
private static final int LOGO_HEIGHT = 80;
public static void main(String[] args) throws Exception {
String text = "https://www.example.com";
String logoPath = "logo.png";
String outputPath = "qrcode_with_logo.png";
// 创建二维码参数
MultiFormatWriter writer = new MultiFormatWriter();
BitMatrix bitMatrix = writer.encode(text, BarcodeFormat.QR_CODE, QRCODE_WIDTH, QRCODE_HEIGHT);
// 加载Logo图片
BufferedImage logoImage = ImageIO.read(new File(logoPath));
// 创建带Logo的二维码
BufferedImage qrcodeImage = new BufferedImage(QRCODE_WIDTH, QRCODE_HEIGHT, BufferedImage.TYPE_INT_RGB);
Graphics2D graphics = (Graphics2D) qrcodeImage.getGraphics();
graphics.drawImage(bitMatrixToImage(bitMatrix), 0, 0, null);
int x = (QRCODE_WIDTH - LOGO_WIDTH) / 2;
int y = (QRCODE_HEIGHT - LOGO_HEIGHT) / 2;
graphics.drawImage(logoImage, x, y, LOGO_WIDTH, LOGO_HEIGHT, null);
graphics.dispose();
// 保存带Logo的二维码图片
ImageIO.write(qrcodeImage, "png", new File(outputPath));
}
private static BufferedImage bitMatrixToImage(BitMatrix matrix) {
int width = matrix.getWidth();
int height = matrix.getHeight();
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
for (int x = 0; x < width; x++) {
for (int y = 0; y < height; y++) {
image.setRGB(x, y, matrix.get(x, y) ? 0xFF000000 : 0xFFFFFFFF);
}
}
return image;
}
}
```
在上面的代码中,我们首先根据给定的URL文本生成了一个普通的二维码。然后,我们加载Logo图片,并使用Graphics2D对象将Logo绘制在二维码中间。最后,将带有Logo的二维码保存为一个新的图片文件。
请确保将`logo.png`替换为你想要添加的Logo图片的路径,并将`outputPath`设置为保存带有Logo的二维码的文件路径。你还可以根据需要调整二维码和Logo的大小。
希望这可以帮助到你!如果还有其他问题,请随时提问。
阅读全文
相关推荐
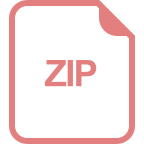
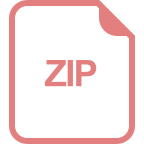
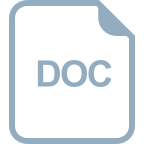
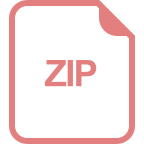
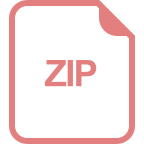
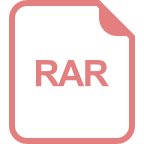
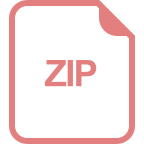
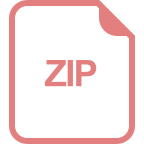
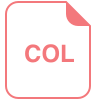
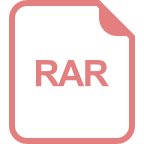