编写一个学生成绩管理程序。建一个单链表,要求能输入和打印学生成绩,并求出所有学生的平均成绩。
时间: 2024-11-12 07:35:46 浏览: 5
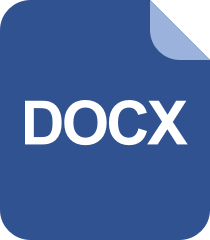
c编写、单链表,对多个班级学生成绩进行管理

编写一个学生成绩管理程序,可以采用结构体或类来表示学生信息,其中包含学生的姓名和成绩。我们可以使用单链表数据结构来存储这些学生的记录,因为插入和删除操作较为方便。
首先,定义一个`Student`节点结构:
```c++
struct Student {
char name[50];
float score;
struct Student* next; // 指向下一个学生的指针
};
```
然后,创建链表相关的函数:
1. `void insertStudent(Student** head, const char* name, float score)`:用于添加新学生到链表头部。
2. `void printStudents(Student* head)`:遍历链表并打印每个学生的名称和分数。
3. `float calculateAverage(Student* head)`:计算链表中所有学生的平均分。
下面是部分关键代码实现:
```c++
// 添加学生
void insertStudent(Student** head, const char* name, float score) {
Student* newNode = (Student*)malloc(sizeof(Student));
newNode->name = (char*)malloc(strlen(name) + 1);
strcpy(newNode->name, name);
newNode->score = score;
newNode->next = *head;
*head = newNode;
}
// 打印学生信息
void printStudents(Student* head) {
if (head == NULL) {
printf("链表为空.\n");
} else {
while (head != NULL) {
printf("姓名: %s, 分数: %.2f\n", head->name, head->score);
head = head->next;
}
}
}
// 计算平均分
float calculateAverage(Student* head) {
float totalScore = 0.0;
int count = 0;
if (head == NULL) {
return 0;
}
Student* current = head;
while (current != NULL) {
totalScore += current->score;
count++;
current = current->next;
}
return totalScore / count;
}
```
要使用这个程序,你可以从用户那里获取输入,然后调用上述函数:
```c
int main() {
Student* head = NULL;
// 输入操作...
// 插入学生...
// 打印学生...
float average = calculateAverage(head);
printf("所有学生的平均成绩: %.2f\n", average);
// 清理内存...
return 0;
}
```
阅读全文
相关推荐
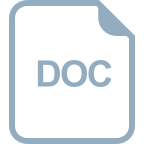
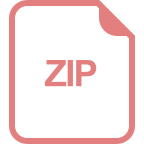















