怎样统计每个单词出现的次数,用c语言解答
时间: 2024-12-23 08:18:06 浏览: 3
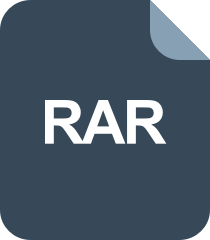
c语言统计.v 文件中的cell数
在C语言中,统计每个单词出现的次数通常需要使用字符串处理、数组或者哈希表(如`struct`结合`hash_map`)。这里是一个基本的步骤说明:
1. **输入阶段**:首先读取输入文本,可以使用标准输入流(stdin)或者文件操作。
2. **分割阶段**:将文本按空格或其他特定分隔符分割成单个单词。这可以用到`strtok`函数,注意要用`strdup`复制每个单词,因为`strtok`会修改原始字符串。
3. **计数阶段**:创建一个结构体或数组,例如`word_count`,其中包含单词作为键,值为该单词的出现次数。对于每个单词,检查它是否已经在字典中,如果存在则增加计数,否则添加新项并将计数设为1。
4. **更新计数**:遍历每个单词并更新相应的计数值。
5. **输出结果**:最后打印出每个单词及其对应的出现次数。
```c
#include <stdio.h>
#include <string.h>
// 使用结构体存储单词计数
typedef struct {
char word[100]; // 假设单词长度不超过100字符
int count;
} WordCount;
int main() {
char input[1000];
fgets(input, sizeof(input), stdin);
input[strcspn(input, "\n")] = '\0'; // 移除行尾换行符
WordCount counts[] = {{"", 0}, {" ", 0}}; // 初始化默认计数
const char *token = strtok(input, " "); // 分割符为" "
while (token != NULL) {
bool found = false;
for (size_t i = 0; i < sizeof(counts) / sizeof(WordCount); ++i) {
if (!strcmp(token, counts[i].word)) {
counts[i].count++;
found = true;
break;
}
}
if (!found) {
// 如果单词未找到,则添加到列表中并设置初始计数为1
strncpy(counts[i + 1].word, token, sizeof(counts[i + 1].word));
counts[i + 1].count = 1;
}
token = strtok(NULL, " ");
}
// 打印结果
for (size_t i = 0; i < sizeof(counts) / sizeof(WordCount); ++i)
printf("%s: %d\n", counts[i].word, counts[i].count);
return 0;
}
```
阅读全文
相关推荐
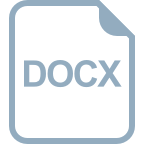
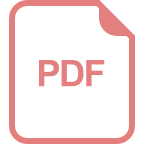


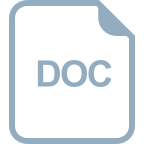
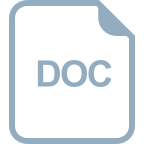
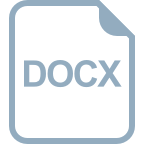
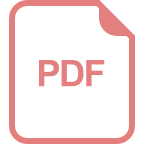
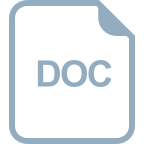
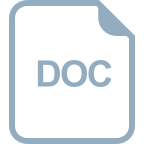
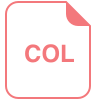

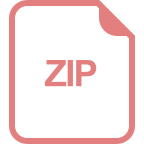
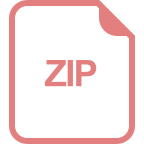
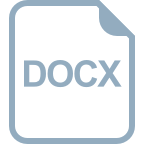