微信小程序获取微信运动步数的实例代码 java
时间: 2023-08-05 08:00:38 浏览: 93
微信小程序是一款非常流行的移动应用程序,它提供了许多功能和API供开发者使用。获取微信运动步数是其中的一个功能,以下是一个使用Java编写的微信小程序获取微信运动步数的实例代码。
```java
import java.net.URL;
import java.net.HttpURLConnection;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.BufferedReader;
public class WeChatMiniProgram {
public static void main(String[] args) {
// 小程序的AppID
String appId = "your_app_id";
// 登录凭证code
String code = "your_code";
// 小程序的AppSecret
String appSecret = "your_app_secret";
// 获取access_token的URL
String accessTokenUrl = "https://api.weixin.qq.com/sns/jscode2session?appid=" + appId + "&secret=" + appSecret + "&grant_type=authorization_code&js_code=" + code;
try {
// 发送HTTP请求获取access_token
URL url = new URL(accessTokenUrl);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
connection.connect();
// 读取返回的数据
InputStream inputStream = connection.getInputStream();
InputStreamReader inputStreamReader = new InputStreamReader(inputStream, "UTF-8");
BufferedReader bufferedReader = new BufferedReader(inputStreamReader);
StringBuilder response = new StringBuilder();
String line;
while ((line = bufferedReader.readLine()) != null) {
response.append(line);
}
// 解析返回的数据
String jsonString = response.toString();
// 提取access_token和openid
String accessToken = // 从jsonString中提取access_token
String openId = // 从jsonString中提取openId
// 获取微信运动步数的URL
String stepCountUrl = "https://api.weixin.qq.com/sns/more_info?access_token=" + accessToken + "&openid=" + openId;
// 发送HTTP请求获取微信运动步数
URL stepUrl = new URL(stepCountUrl);
HttpURLConnection stepConnection = (HttpURLConnection) stepUrl.openConnection();
stepConnection.setRequestMethod("GET");
stepConnection.connect();
// 读取返回的数据
InputStream stepInputStream = stepConnection.getInputStream();
InputStreamReader stepInputStreamReader = new InputStreamReader(stepInputStream, "UTF-8");
BufferedReader stepBufferedReader = new BufferedReader(stepInputStreamReader);
StringBuilder stepResponse = new StringBuilder();
String stepLine;
while ((stepLine = stepBufferedReader.readLine()) != null) {
stepResponse.append(stepLine);
}
// 解析返回的数据
String stepJsonString = stepResponse.toString();
// 提取微信运动步数
int stepCount = // 从stepJsonString中提取微信运动步数
// 输出微信运动步数
System.out.println("微信运动步数:" + stepCount);
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
以上代码中,通过发送HTTP请求获取微信小程序的`access_token`和`openId`,然后使用`access_token`和`openId`来发送第二个HTTP请求,获取微信运动步数。需要注意的是,你需要将代码中的`your_app_id`、`your_code`和`your_app_secret`替换为你自己的实际值。此外,代码中的JSON解析部分需要进行具体的实现。
相关推荐
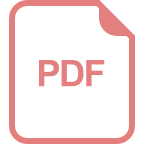















