python 写入文件是在字符串后面添加空格
时间: 2023-09-01 17:02:03 浏览: 299
Python写入文件时,在字符串后面添加空格可以通过以下几种方式实现。
1.使用字符串连接操作符`+`,将空格字符追加到需要写入的字符串末尾。例如:
```
text = "Hello World"
text += " " # 在字符串后面添加一个空格
with open("output.txt", "w") as file:
file.write(text)
```
2.使用字符串的`join`方法,将需要写入的字符串和空格字符以列表形式传入,并使用空格字符作为分隔符进行连接。例如:
```
text = "Hello World"
space = " "
text_with_space = space.join([text, space]) # 在字符串后面添加一个空格
with open("output.txt", "w") as file:
file.write(text_with_space)
```
3.使用字符串的格式化操作符`%`,将需要写入的字符串和空格字符格式化为一个新的字符串,再进行写入操作。例如:
```
text = "Hello World"
text_with_space = "%s " % text # 在字符串后面添加一个空格
with open("output.txt", "w") as file:
file.write(text_with_space)
```
无论使用哪种方式,在写入文件时都需要明确指定文件的打开模式为写入模式("w")。
相关问题
python 读取csv 字符串有多余空格
在Python中读取CSV文件时,有时会遇到字符串中存在多余空格的情况。为了解决这个问题,可以采取以下几种方法:
1. 使用strip()函数去除字符串两端的空格。在读取CSV文件时,可以使用strip()函数对每个字符串进行处理,去除多余的空格。
2. 使用pandas库的str.strip()函数。如果使用pandas库读取CSV文件,可以使用str.strip()函数对字符串进行处理,去除多余的空格。
3. 在写入CSV文件时,使用参数设置newline=""。在写入CSV文件时,可以在打开文件时添加参数newline="",这样可以去除多余的空行。
综上所述,可以通过以上方法来解决Python读取CSV文件时字符串存在多余空格的问题。
#### 引用[.reference_title]
- *1* *3* [Python写入CSV出现空行解决方法](https://blog.csdn.net/ghaokl/article/details/128962771)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^koosearch_v1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* [Python:读取CSV文件的某几列](https://blog.csdn.net/wenkao123456/article/details/105303269)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^koosearch_v1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
python 读取csv 字符串有多余空格删不掉
要删除读取的CSV文件中的多余空格,可以使用strip()函数来去除字符串两端的空格。在读取CSV文件时,可以使用pandas库的read_csv函数来读取文件,并使用strip()函数对字符串进行处理。以下是修正后的代码示例:
```python
import pandas as pd
data = pd.read_csv('filename.csv')
data = data.apply(lambda x: x.str.strip() if x.dtype == "object" else x)
print(data)
```
这段代码使用pandas库的read_csv函数读取CSV文件,并使用apply函数对每一列进行处理。如果列的数据类型是字符串(object),则使用strip()函数去除字符串两端的空格。最后打印处理后的数据。这样就可以删除读取的CSV文件中的多余空格了。\[3\]
#### 引用[.reference_title]
- *1* *2* [Python写入CSV出现空行解决方法](https://blog.csdn.net/ghaokl/article/details/128962771)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^koosearch_v1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *3* [使用python处理csv文件(读取、删除、替换)](https://blog.csdn.net/zhulewen/article/details/127947531)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^koosearch_v1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
相关推荐
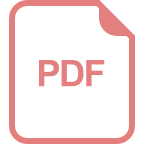
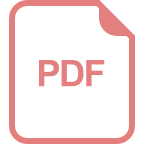
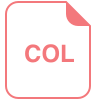
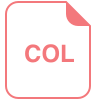
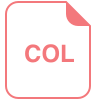
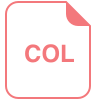
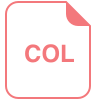







