arm sd 挂载并且写入C 程序
时间: 2024-10-01 22:05:18 浏览: 37
ARM SD卡通常用于嵌入式系统中,如 Raspberry Pi 或者其他的单板电脑。挂载SD卡并从上面读取或写入数据在C程序中需要几个步骤:
1. **安装必要的库**:首先,确保你的系统已经安装了文件系统操作相关的库,例如`mtd`(Memory Technology Device)驱动和`fatfs`库,它们常用于处理SD卡。
2. **初始化MTD设备**:你需要通过系统API找到对应SD卡的MTD设备,并初始化它。这通常涉及到打开设备文件、读取分区表等。
3. **挂载文件系统**:确认MTD设备后,你可以将SD卡的MTD分区挂载到Linux的文件系统中,比如ext4、FAT32等,这一步会创建一个虚拟的文件系统路径供后续操作。
4. **打开文件和写入**:使用`open()`函数打开你想要写入的文件,然后使用`write()`或`fprintf()`等函数写入数据。记得关闭文件句柄以释放资源。
5. **示例代码片段**:
```c
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#include <linux/mtd.h>
#include <sys/mtd/mtd.h>
#include <sys/mount.h>
#include "fsl_fatfs.h"
int main() {
int mtd_fd = open("/dev/mtdblock0", O_RDWR); // 打开MTD设备
if (mtd_fd < 0) {
perror("Failed to open MTD device");
return -1;
}
// 检查并加载文件系统
struct mtd_info *mtd_info = get_mtd_device_by_name("your_device_name"); // 替换为你设备的实际名称
if (!mtd_info) {
perror("Failed to find MTD device");
close(mtd_fd);
return -1;
}
// 挂载文件系统
char mount_point[] = "/mnt/sdcard";
if (mkdir(mount_point, 0755) != 0) {
perror("Failed to create mount point");
close(mtd_fd);
return -1;
}
if (fatfs_mount(mtd_info->name, mount_point, &FatFs) != FR_OK) {
perror("Failed to mount FATFS");
rmdir(mount_point);
close(mtd_fd);
return -1;
}
// 写入文件
char filename[] = "test.txt";
int file_fd = open((mount_point "/" filename), O_WRONLY | O_CREAT, S_IRUSR | S_IWUSR);
if (file_fd < 0) {
perror("Failed to open file");
fatfs_unmount();
close(mtd_fd);
return -1;
}
fprintf(file_fd, "Hello from C program\n");
close(file_fd);
// 卸载文件系统
fatfs_unmount();
close(mtd_fd);
return 0;
}
```
阅读全文
相关推荐
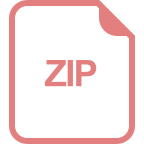
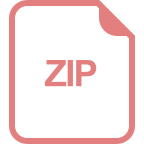
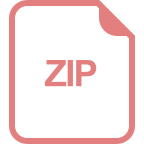
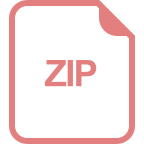
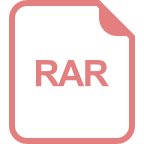
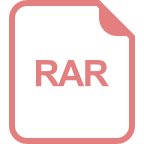
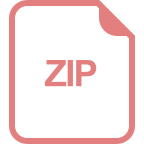
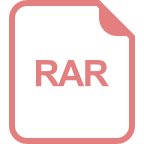
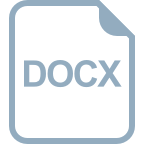
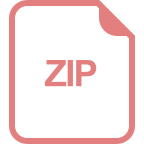
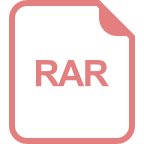
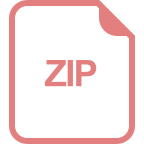
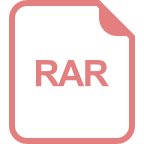
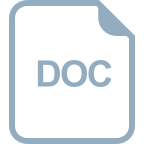
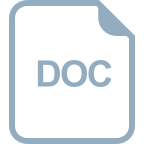
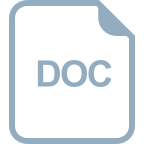
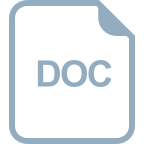
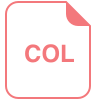

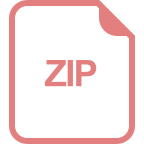