设计一个程序判断输入的一个坐标点是否在一个以坐标轴原点为中心,以 x轴与y轴为对称轴的六边形范围内,六边形边长为1
时间: 2024-10-25 10:11:53 浏览: 12
要设计一个程序判断一个坐标点是否在一个以原点为中心,以x轴和y轴为对称轴的六边形内(每个顶点到原点的距离都是1),你可以使用勾股定理来计算该点到各个对称轴的距离,然后检查这些距离是否满足六边形的条件。
六边形有六个顶点,分别是:
- (1,0) (顺时针)
- (-1,0)
- (0,1)
- (0,-1)
- (1/sqrt(3), 2*sqrt(3)/3) (在第一象限的顶点)
- (1/sqrt(3), -2*sqrt(3)/3)
对于任意给定点(x, y),你需要比较以下距离:
1. 到x轴的距离 |y| 是否小于等于1?
2. 到y轴的距离 |x| 是否小于等于1?
3. 到点(-1, 0)、(1, 0) 和 (0, -1) 的距离是否都小于或等于1?这些点构成了一条边,所以直接计算两点间的距离即可。
以下是一个简单的C++代码实现:
```cpp
#include <cmath>
#include <iostream>
bool isInsideHexagon(double x, double y) {
if (std::abs(y) <= 1 && std::abs(x) <= 1) { // 判断在x轴和y轴上
return true;
}
double distance_to_x_axis = std::abs(y);
double distance_to_y_axis = std::abs(x);
// 检查到其他顶点的距离
double distance_to_other_vertices[6] = {
std::hypot(x + 1, y),
std::hypot(x - 1, y),
std::hypot(x, y + 1),
std::hypot(x, y - 1),
std::hypot(x + 1/sqrt(3), y + 2*sqrt(3)/3),
std::hypot(x + 1/sqrt(3), y - 2*sqrt(3)/3)
};
for (double d : distance_to_other_vertices) {
if (d > 1) {
return false; // 如果任何一边超出范围,则不在六边形内
}
}
return true;
}
int main() {
double coord_x, coord_y;
std::cout << "Enter the coordinates (x, y): ";
std::cin >> coord_x >> coord_y;
if (isInsideHexagon(coord_x, coord_y)) {
std::cout << "The point is inside the hexagon.\n";
} else {
std::cout << "The point is outside the hexagon.\n";
}
return 0;
}
```
阅读全文
相关推荐
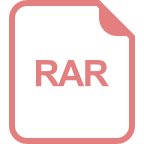
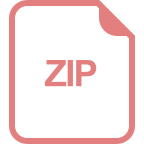
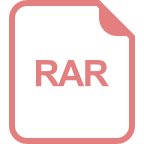
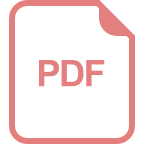
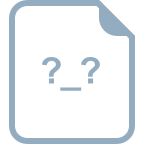
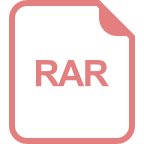
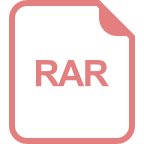
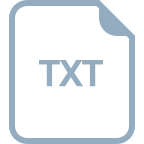
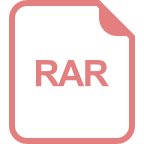
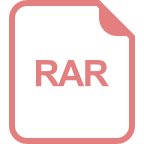
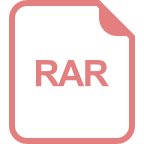
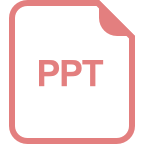
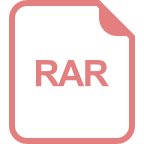
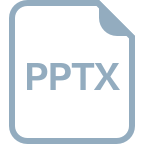
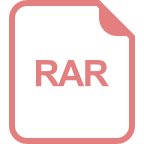
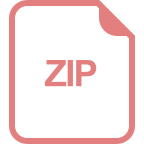
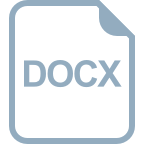
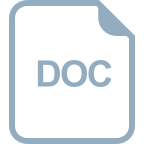
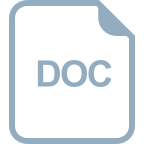