dfs算法路径规划python
时间: 2024-05-17 16:09:36 浏览: 205
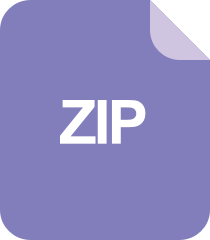
基于深度优先搜索(DFS)的路径规划算法(Python实现)
DFS(深度优先搜索)是一种常用的图遍历算法,用于寻找图中的路径。在路径规划中,DFS可以用来找到从起点到目标点的一条路径。
以下是DFS算法路径规划的Python实现:
```python
def dfs(graph, start, end, path=[]):
path = path + [start]
if start == end:
return path
if start not in graph:
return None
for node in graph[start]:
if node not in path:
new_path = dfs(graph, node, end, path)
if new_path:
return new_path
return None
```
上述代码中,`graph`表示图的邻接表,`start`表示起点,`end`表示目标点,`path`表示当前已经走过的路径。函数首先将起点加入路径中,然后判断是否到达目标点,如果是则返回路径。如果起点不在图中,则返回None。否则,遍历起点的邻居节点,如果邻居节点不在当前路径中,则递归调用DFS函数继续搜索。如果找到一条路径,则返回该路径;否则返回None。
阅读全文
相关推荐
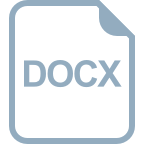
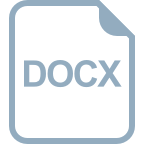















