c++利用递归和分治策略,写出可生成n个字符的全排列的程序
时间: 2024-11-09 11:17:32 浏览: 23
在 C++ 中,我们可以使用递归和分治策略来编写一个生成 n 个字符全排列的程序。这里我们先假设所有字符都是唯一的,例如小写字母 'a' 到 'z'。以下是一个简单的示例:
```cpp
#include <iostream>
#include <string>
// 辅助函数,用于递归生成排列
void permute(std::string str, int start, std::string &result) {
if (start == str.length()) {
result.append(str);
std::cout << "Generated permutation: " << result << std::endl;
} else {
// 对每个未选择的位置,递归地添加下一个字符
for (int i = start; i < str.length(); ++i) {
std::swap(str[start], str[i]); // 交换位置
permute(str, start + 1, result); // 继续递归
std::swap(str[start], str[i]); // 撤销交换,恢复原状态
}
}
}
// 主函数
int main() {
int n;
std::cout << "Enter the number of characters (n): ";
std::cin >> n;
// 创建一个包含所有可能字符的小字符串
std::string chars = "abcdefghijklmnopqrstuvwxyz";
std::string current_permutation;
permute(chars, 0, current_permutation); // 开始生成
return 0;
}
```
这个程序首先读取用户输入的字符数量 `n`,然后创建一个包含所有可能字符的字符串。`permute` 函数是一个递归函数,它从第一个字符开始,尝试将其放在所有尚未考虑的位置上,并对剩余的字符继续进行同样的操作。当所有字符都已放置好后,就生成了一个排列。
阅读全文
相关推荐
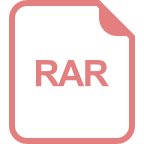
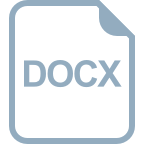
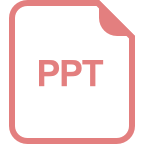
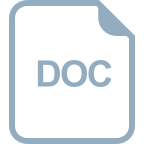
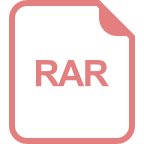
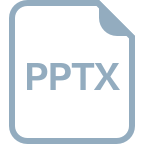
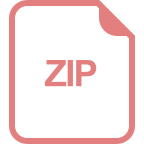
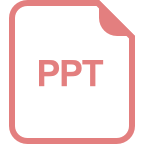
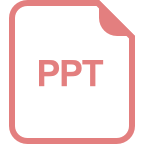
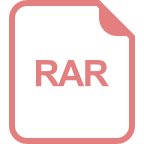
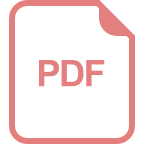
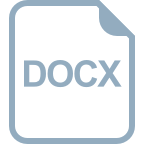
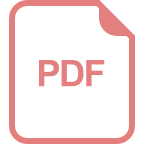
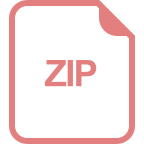