卷积神经网络代码tensorflow
时间: 2023-11-01 08:07:04 浏览: 111
当然,我可以帮你提供一个简单的卷积神经网络的代码示例。以下是一个使用TensorFlow库的基本卷积神经网络的示例代码:
```python
import tensorflow as tf
# 创建卷积神经网络模型
def create_model():
model = tf.keras.models.Sequential([
tf.keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)),
tf.keras.layers.MaxPooling2D((2, 2)),
tf.keras.layers.Flatten(),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
return model
# 加载MNIST数据集并进行预处理
(x_train, y_train), (x_test, y_test) = tf.keras.datasets.mnist.load_data()
x_train = x_train.reshape(-1, 28, 28, 1).astype('float32') / 255.0
x_test = x_test.reshape(-1, 28, 28, 1).astype('float32') / 255.0
y_train = tf.keras.utils.to_categorical(y_train)
y_test = tf.keras.utils.to_categorical(y_test)
# 创建模型实例
model = create_model()
# 编译模型
model.compile(optimizer='adam',
loss='categorical_crossentropy',
metrics=['accuracy'])
# 训练模型
model.fit(x_train, y_train, epochs=5, batch_size=64, validation_data=(x_test, y_test))
# 使用测试集评估模型性能
test_loss, test_acc = model.evaluate(x_test, y_test)
print('Test accuracy:', test_acc)
```
上述代码使用了`tf.keras`库来构建卷积神经网络模型,并使用MNIST数据集进行训练和测试。模型包含一个卷积层、一个池化层、一个展平层、两个全连接层以及一个输出层。代码中的`create_model`函数用于创建模型实例,通过编译模型并使用训练数据进行训练,最后使用测试数据评估模型的性能。
请注意,在实际应用中,您可能需要根据您的问题和数据来调整模型的结构和超参数。希望这个简单的示例能够帮助您入门卷积神经网络的编程实践!
阅读全文
相关推荐
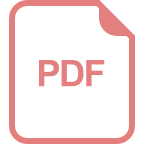
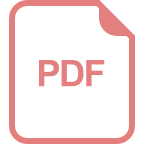
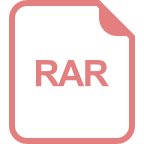
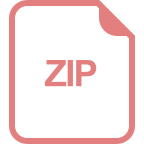
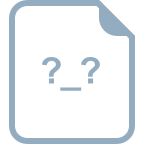
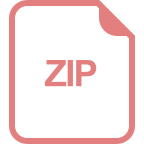
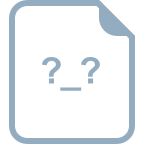
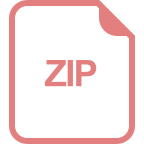
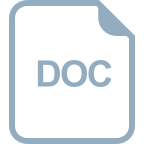
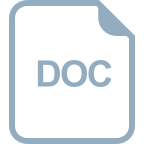
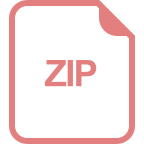
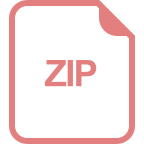
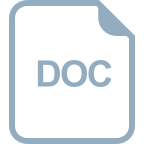
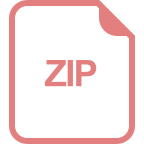
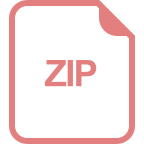
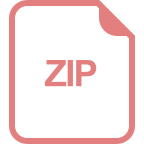
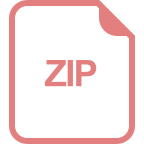