lombook 有参无参构造
时间: 2023-07-30 22:11:23 浏览: 59
Lombok 是一个 Java 库,它提供了一些注解来简化 Java 代码的编写。其中,@NoArgsConstructor 和 @AllArgsConstructor 注解用于生成无参和全参构造方法。
使用 @NoArgsConstructor 注解可以在类上自动生成一个无参构造方法,示例如下:
```java
import lombok.NoArgsConstructor;
@NoArgsConstructor
public class MyClass {
// 类的成员变量和方法
}
```
使用 @AllArgsConstructor 注解可以在类上自动生成一个包含所有成员变量的全参构造方法,示例如下:
```java
import lombok.AllArgsConstructor;
@AllArgsConstructor
public class MyClass {
private int id;
private String name;
// 其他成员变量和方法
}
```
通过使用这两个注解,我们可以省去手动编写构造方法的麻烦,并且减少了一些重复劳动。
相关问题
lombook 构造方法
Lombok 是一个 Java 开发的实用工具库,它可以通过注解来简化代码的编写,其中包括了自动生成构造方法的功能。
使用 Lombok 来自动生成构造方法非常简单,只需要在类上添加 `@AllArgsConstructor` 注解即可生成一个包含所有字段的全参构造方法。例如:
```java
import lombok.AllArgsConstructor;
@AllArgsConstructor
public class MyClass {
private String field1;
private int field2;
// 此处会自动生成一个包含所有字段的构造方法
}
```
除了 `@AllArgsConstructor`,Lombok 还提供了其他注解来生成不同类型的构造方法,比如 `@NoArgsConstructor` 可以生成无参构造方法,`@RequiredArgsConstructor` 可以生成带有必需字段的构造方法等等。
需要注意的是,在使用 Lombok 自动生成构造方法时,确保已经正确安装并配置了 Lombok 插件,以便在编译时生成对应的构造方法代码。
lombook SneakyThrows
Lombok's `@SneakyThrows` annotation is used to suppress checked exceptions without explicitly declaring them in the method signature. It allows you to throw checked exceptions without adding them to the method signature or handling them with a try-catch block.
By adding `@SneakyThrows` to a method, Lombok automatically wraps any checked exception thrown within that method and rethrows it as a runtime exception. This simplifies the code and avoids the need for unnecessary try-catch blocks.
Here's an example of how to use `@SneakyThrows`:
```java
import lombok.SneakyThrows;
public class Example {
@SneakyThrows
public void doSomething() {
// code that throws a checked exception
}
}
```
In this example, if the code inside the `doSomething` method throws a checked exception, it will be wrapped in a `RuntimeException` and propagated without requiring the method to declare or handle the exception explicitly.
相关推荐
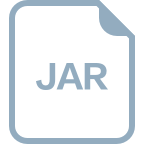











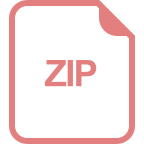