c语言创建一个包含学生基本信息(学号、姓名、性别、专业、成绩)的线性链表,
时间: 2024-09-11 14:12:36 浏览: 93
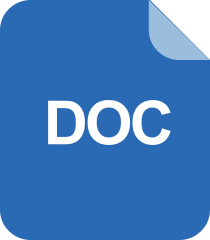
学生成绩管理系统程序设计
在C语言中创建一个包含学生基本信息的线性链表,首先需要定义学生信息的结构体,然后创建链表节点,并通过指针将各个节点链接起来。下面是一个简单的示例实现:
1. 定义学生信息结构体:
```c
typedef struct Student {
int id; // 学号
char name[50]; // 姓名
char gender[10]; // 性别
char major[50]; // 专业
float score; // 成绩
} Student;
```
2. 创建链表节点:
```c
typedef struct Node {
Student data; // 学生信息
struct Node* next; // 指向下一个节点的指针
} Node;
```
3. 初始化链表和添加学生信息节点:
```c
Node* createList() {
Node* head = (Node*)malloc(sizeof(Node)); // 创建头节点
if (head == NULL) {
perror("Unable to allocate memory");
exit(1);
}
head->next = NULL; // 初始化为空链表
// 添加学生信息
// 假设有一个学生信息数组,这里简化为手动添加
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data.id = 1;
strcpy(newNode->data.name, "张三");
strcpy(newNode->data.gender, "男");
strcpy(newNode->data.major, "计算机科学与技术");
newNode->data.score = 95.5;
newNode->next = head->next; // 将新节点链接到头节点之后
head->next = newNode; // 更新头节点的next指针
return head; // 返回头节点
}
```
4. 遍历打印链表中的学生信息:
```c
void printList(Node* head) {
Node* current = head->next; // 从头节点的下一个节点开始
while (current != NULL) {
printf("学号:%d, 姓名:%s, 性别:%s, 专业:%s, 成绩:%.1f\n",
current->data.id, current->data.name, current->data.gender, current->data.major, current->data.score);
current = current->next; // 移动到下一个节点
}
}
```
5. 释放链表内存:
```c
void freeList(Node* head) {
Node* current = head;
Node* next;
while (current != NULL) {
next = current->next;
free(current);
current = next;
}
}
```
使用这些函数,你可以创建一个包含学生基本信息的线性链表,并进行打印、添加新节点、释放内存等操作。
阅读全文
相关推荐
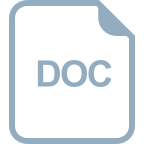
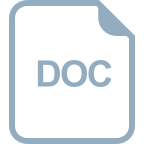
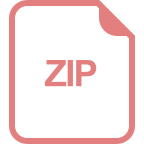
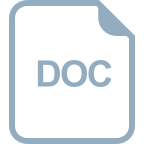
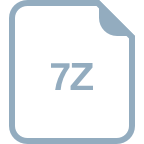
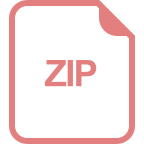
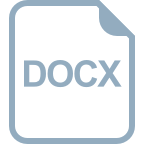
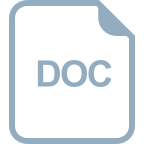
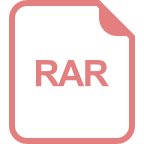
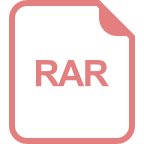
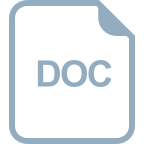
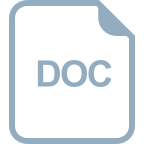
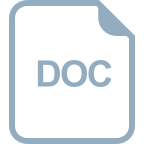
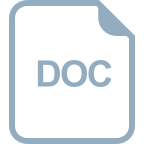
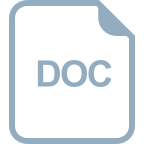
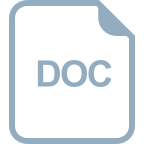
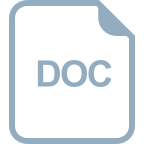
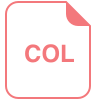