java 如何用注解aop拦截controller 所有方法
时间: 2023-08-09 20:01:40 浏览: 92
在Java中,可以使用注解和AOP(面向切面编程)技术来拦截Controller的所有方法。
首先,需要创建一个自定义的注解,用于标识需要拦截的方法。可以使用如下的注解定义:
```java
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface Intercept {
}
```
接下来,创建一个切面类,用于实现拦截逻辑。可以使用如下的切面类定义:
```java
import org.aspectj.lang.annotation.After;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.aspectj.lang.annotation.Pointcut;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class ControllerInterceptor {
@Pointcut("@annotation(Intercept)")
public void interceptedMethods() {}
@Before("interceptedMethods()")
public void beforeIntercept() {
// 在方法执行之前拦截的逻辑
}
@After("interceptedMethods()")
public void afterIntercept() {
// 在方法执行之后拦截的逻辑
}
}
```
在上述的切面类中,使用了`@Aspect`注解表示这是一个切面类,使用了`@Component`注解将切面类交由Spring管理。`@Pointcut`注解定义了需要拦截的方法,此处使用了`@annotation(Intercept)`表示拦截带有`Intercept`注解的方法。`@Before`和`@After`注解分别表示在方法执行前和执行后进行拦截处理。
最后,在需要拦截的Controller的方法上使用`@Intercept`注解进行标记,例如:
```java
@RestController
public class MyController {
@Intercept
@GetMapping("/")
public String index() {
return "Hello World";
}
}
```
这样,只要在Controller的方法上使用了`@Intercept`注解,就会触发切面类中的拦截逻辑。
需要注意的是,上述代码使用了Spring AOP来实现AOP功能,因此需要在Spring配置文件中开启AOP的支持。另外,还需要引入相关的依赖,例如Spring AOP的依赖。
相关推荐
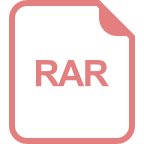
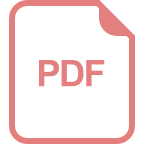
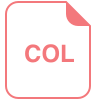
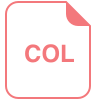
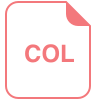
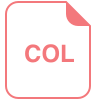
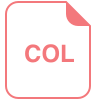









