用c语言实现 du -sh命令
时间: 2023-10-19 07:25:50 浏览: 142
以下是使用C语言实现du -sh命令的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/stat.h>
long long calculateSize(const char *path) {
struct stat st;
if (stat(path, &st) == 0) {
if (S_ISDIR(st.st_mode)) {
long long totalSize = 0;
DIR *dir = opendir(path);
struct dirent *dp;
while ((dp = readdir(dir)) != NULL) {
if (strcmp(dp->d_name, ".") != 0 && strcmp(dp->d_name, "..") != 0) {
char childPath[1024];
snprintf(childPath, sizeof(childPath), "%s/%s", path, dp->d_name);
totalSize += calculateSize(childPath);
}
}
closedir(dir);
return totalSize;
} else if (S_ISREG(st.st_mode)) {
return st.st_size;
}
}
return 0;
}
void printHumanReadableSize(long long size) {
const char *units[] = {"B", "KB", "MB", "GB", "TB"};
int unitIndex = 0;
while (size >= 1024 && unitIndex < sizeof(units) / sizeof(units[0])) {
size /= 1024;
unitIndex++;
}
printf("%lld%s\n", size, units[unitIndex]);
}
int main(int argc, char *argv[]) {
if (argc != 2) {
printf("Usage: %s <directory_path>\n", argv[0]);
return 1;
}
const char *path = argv[1];
long long totalSize = calculateSize(path);
printf("Total Size: ");
printHumanReadableSize(totalSize);
return 0;
}
```
这个程序会计算指定路径下文件夹的总大小,并以人类可读的格式打印出来。请注意,这只是一个简单的示例,可能不支持符号链接和特殊文件等。
阅读全文
相关推荐
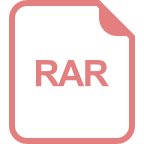
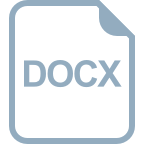
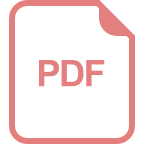
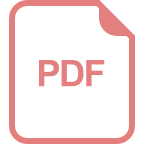
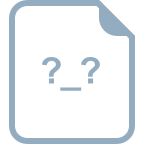
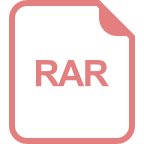
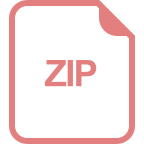
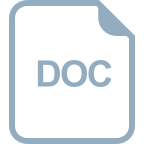
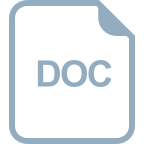
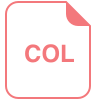
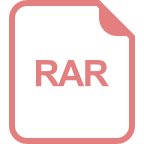
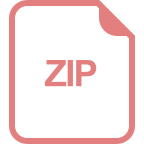
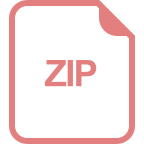
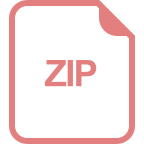
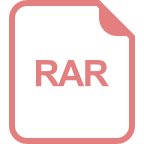
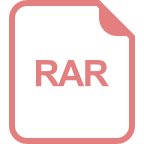
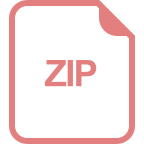
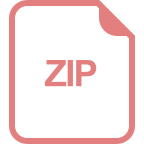