如何以c++写一个以.txt文件中的单词为词库的随机单词生成器?
时间: 2024-10-06 15:01:35 浏览: 42
在C++中,你可以使用`fstream`库来操作文本文件,并从里面读取单词作为词库。以下是一个简单的步骤:
1. 包含头文件:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <cstdlib> // 引入random库用于随机数生成
#include <ctime> // 获取当前时间作为随机数种子
```
2. 定义一个函数来读取文件并存储单词到一个容器(如vector)中:
```cpp
std::vector<std::string> readWordsFromFile(const std::string& filename) {
std::ifstream file(filename);
if (!file.is_open()) {
std::cerr << "Failed to open the file." << std::endl;
return {};
}
std::vector<std::string> words;
std::string word;
while (std::getline(file, word)) {
words.push_back(word);
}
file.close();
return words;
}
```
3. 写一个函数来生成随机单词:
```cpp
std::string getRandomWord(const std::vector<std::string>& wordList) {
srand(time(0)); // 设置随机数种子
int randomIndex = rand() % wordList.size(); // 生成0到词库大小减一的随机数
return wordList[randomIndex];
}
```
4. 主函数中调用这两个函数,生成并输出随机单词:
```cpp
int main() {
std::vector<std::string> wordList = readWordsFromFile("wordlist.txt");
if (wordList.empty()) {
return 1; // 文件为空或无法打开
}
std::cout << "Random Word: " << getRandomWord(wordList) << std::endl;
return 0;
}
```
记得将上述代码中的"wordlist.txt"替换为实际包含单词的文本文件路径。
阅读全文
相关推荐
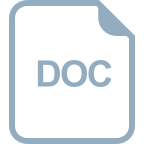
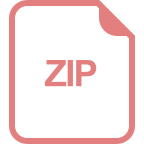
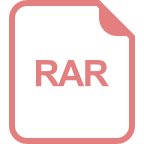
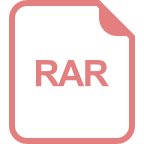
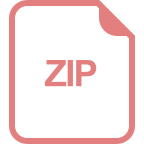
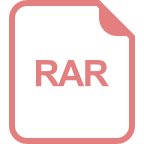
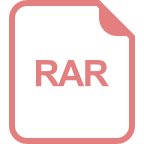
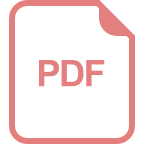
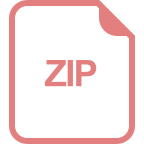
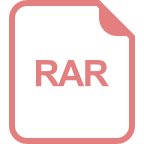
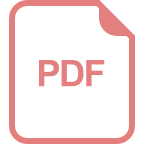
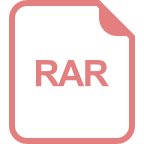