颜色直方图python
时间: 2023-11-11 07:00:42 浏览: 204
要绘制颜色直方图,可以使用Python中的matplotlib库。以下是一个简单的示例代码:
```python
import cv2
import matplotlib.pyplot as plt
# 读取图像
img = cv2.imread('image.jpg')
# 将图像转换为RGB格式
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
# 计算颜色直方图
hist, bins = plt.hist(img.ravel(), bins=256, range=[0, 256])
# 绘制直方图
plt.plot(hist)
# 显示图像和直方图
plt.imshow(img)
plt.show()
```
相关问题
颜色直方图python代码
下面是一个计算图像颜色直方图的简单 Python 代码示例:
```python
import cv2
import matplotlib.pyplot as plt
def plot_color_histogram(image_path):
# 读取图像
image = cv2.imread(image_path)
# 将图像转换为 RGB 格式
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
# 计算直方图
histogram = cv2.calcHist([image], [0, 1, 2], None, [256, 256, 256], [0, 256, 0, 256, 0, 256])
# 绘制直方图
plt.figure()
plt.title('Color Histogram')
plt.xlabel('Bins')
plt.ylabel('# of Pixels')
plt.imshow(histogram, interpolation='nearest')
plt.show()
# 调用函数并传入图像路径
plot_color_histogram('image.jpg')
```
注意,这里使用了 OpenCV (`cv2`) 库来读取和处理图像,同时还使用了 Matplotlib (`plt`) 库来绘制直方图。您需要确保已经安装了这两个库。
图像特征颜色直方图python
在Python中,可以使用OpenCV库来计算图像的颜色直方图。以下是一个简单的示例代码:
```python
import cv2
import matplotlib.pyplot as plt
# 读取图像
img = cv2.imread('image.jpg')
# 将图像转换为HSV颜色空间
hsv_img = cv2.cvtColor(img, cv2.COLOR_BGR2HSV)
# 计算直方图
hist = cv2.calcHist([hsv_img], [0, 1], None, [180, 256], [0, 180, 0, 256])
# 显示直方图
plt.imshow(hist, interpolation='nearest')
plt.show()
```
这段代码将读取名为'image.jpg'的图像,将其转换为HSV颜色空间,并计算其颜色直方图。最后,使用Matplotlib库显示直方图。
阅读全文
相关推荐
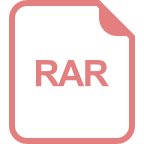
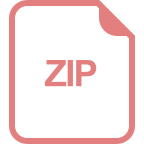
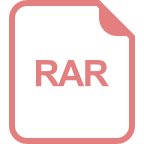













