C++语言,读到文件末尾则返回值
时间: 2024-10-10 18:06:52 浏览: 26
在C++中,当你读取文件时,通常会通过检测是否达到文件的结束标记(`std::ios_base::eof()`)来判断是否到了文件的末尾。你可以结合`std::ifstream`流的成员函数如`getline()`或`read()`来检查。如果达到了文件末尾,这些函数通常不会抛出异常,而是返回一个特定的状态。
以下是一个示例,展示了如何在读取文件时检查是否到达末尾并返回相应的结果:
```cpp
#include <fstream>
#include <string>
bool readToEndOfFile(std::ifstream& file, std::string& content) {
if (!file.is_open()) {
std::cerr << "Failed to open the file." << std::endl;
return false;
}
char buffer[4096]; // 假设最大行长度为4K字节
std::string line;
while (file >> std::noskipws >> line) { // 逐行读取,跳过空格
content += line + "\n"; // 添加到结果字符串
if (file.peek() == EOF) { // 当前字符是文件末尾
break; // 结束读取
}
}
file.clear(); // 清除输入流中的错误标志
file.seekg(0, std::ios::end); // 移动到文件末尾
if (file.tellg() == 0) { // 检查是否真的到了末尾
file.close();
return true; // 成功读到末尾
} else {
file.close();
return false; // 文件未完全读完
}
}
int main() {
std::string content;
std::ifstream file("your_file.txt");
bool reachedEnd = readToEndOfFile(file, content);
if (reachedEnd) {
std::cout << "Successfully read till end of file: " << content << std::endl;
} else {
std::cerr << "Did not reach the end of the file." << std::endl;
}
return 0;
}
```
在上面的代码中,`readToEndOfFile`函数会持续读取文件,直到遇到文件末尾或无法再读取新行。然后它会关闭文件并根据文件指针的位置决定是否到达了文件末尾。
阅读全文
相关推荐
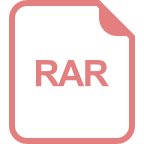
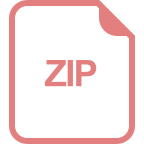
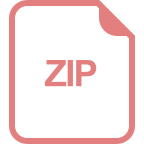
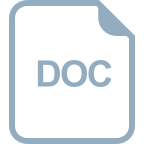
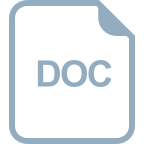
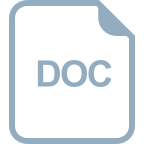
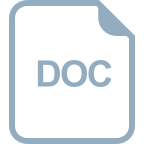
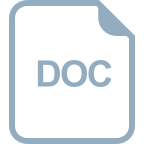
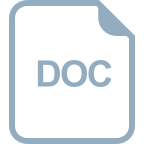






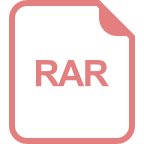
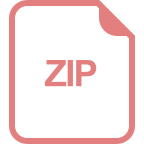