how to convert doc to docx using poi?
时间: 2024-04-10 11:34:03 浏览: 483
To convert a DOC file to DOCX format using Apache POI library, you can follow these steps:
1. Add the Apache POI dependency to your project. You can do this by adding the necessary JAR files to your project's build path or by using a dependency management tool like Maven or Gradle.
2. Use the following code snippet to perform the conversion:
```java
import org.apache.poi.hwpf.HWPFDocument;
import org.apache.poi.hwpf.converter.WordToConverter;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.InputStream;
import java.io.OutputStream;
public class DocToDocxConverter {
public static void main(String[] args) {
try {
// Load the DOC file
InputStream inputStream = new FileInputStream("input.doc");
HWPFDocument document = new HWPFDocument(inputStream);
// Create an empty output DOCX file
OutputStream outputStream = new FileOutputStream("output.docx");
XWPFDocument convertedDocument = new XWPFDocument();
// Convert the DOC file to DOCX format
WordToConverter converter = new WordToConverter(convertedDocument);
converter.processDocument(document);
// Save the converted document to the output file
convertedDocument.write(outputStream);
// Close the streams
outputStream.close();
inputStream.close();
System.out.println("Conversion completed successfully.");
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
Make sure to replace "input.doc" with the path to your input DOC file and "output.docx" with the desired path for the output DOCX file.
3. Run the code, and it will convert the DOC file to DOCX format and save it as "output.docx" in the specified location.
Please note that this code is based on Apache POI version 5.x, which supports the conversion of DOC to DOCX. If you are using an older version of Apache POI, you might need to use different classes or methods for the conversion.
阅读全文
相关推荐





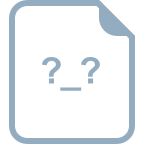










