stm32f103c8t6实现简易计算器
时间: 2023-11-14 11:08:00 浏览: 139
以下是基于STM32F103C8T6的简易计算器的实现方法:
1. 首先需要连接5110屏幕和4*4键盘到STM32F103C8T6开发板上。
2. 然后需要编写程序,实现基本的多位加减乘除功能。可以使用C语言编写程序,并使用Keil或者其他编译器进行编译。
3. 在程序中需要定义键盘输入的方式,并将输入的数字和运算符存储在变量中。
4. 然后需要编写算法,根据输入的数字和运算符进行计算,并将结果显示在5110屏幕上。
5. 最后需要测试程序,确保计算器能够正确地进行加减乘除运算。
以下是一个基于STM32F103C8T6的简易计算器的代码示例:
```c
#include "stm32f10x.h"
#include "stdio.h"
#include "stdlib.h"
#include "string.h"
#define ROW1 GPIO_Pin_0
#define ROW2 GPIO_Pin_1
#define ROW3 GPIO_Pin_2
#define ROW4 GPIO_Pin_3
#define COL1 GPIO_Pin_4
#define COL2 GPIO_Pin_5
#define COL3 GPIO_Pin_6
#define COL4 GPIO_Pin_7
void delay_ms(uint16_t nms)
{
uint32_t i;
for(i=0;i<nms*1000;i++);
}
void GPIO_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA|RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = ROW1|ROW2|ROW3|ROW4;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = COL1|COL2|COL3|COL4; GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
}
void LCD5110_GPIO_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_1|GPIO_Pin_2|GPIO_Pin_3|GPIO_Pin_4|GPIO_Pin_5;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_OD;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
}
void LCD5110_WriteByte(uint8_t data,uint8_t command)
{
uint8_t i;
GPIO_ResetBits(GPIOA,GPIO_Pin_0);
if(command==0)
GPIO_SetBits(GPIOA,GPIO_Pin_1);
else
GPIO_ResetBits(GPIOA,GPIO_Pin_1);
for(i=0;i<8;i++)
{
if(data&0x80)
GPIO_SetBits(GPIOA,GPIO_Pin_5);
else
GPIO_ResetBits(GPIOA,GPIO_Pin_5);
GPIO_SetBits(GPIOA,GPIO_Pin_2);
GPIO_ResetBits(GPIOA,GPIO_Pin_2);
data<<=1;
}
}
void LCD5110_Init(void)
{
GPIO_SetBits(GPIOA,GPIO_Pin_0);
delay_ms(10);
GPIO_ResetBits(GPIOA,GPIO_Pin_0);
delay_ms(10);
GPIO_SetBits(GPIOA,GPIO_Pin_0);
LCD5110_WriteByte(0x21,1);
LCD5110_WriteByte(0xc8,1);
LCD5110_WriteByte(0x06,1);
LCD5110_WriteByte(0x13,1);
LCD5110_WriteByte(0x20,1);
LCD5110_WriteByte(0x0c,1);
}
void LCD5110_Clear(void)
{
uint16_t i;
for(i=0;i<504;i++)
LCD5110_WriteByte(0,0);
}
void LCD5110_Set_XY(uint8_t X,uint8_t Y)
{
LCD5110_WriteByte(0x40|Y,1);
LCD5110_WriteByte(0x80|X,1);
}
void LCD5110_Write_Char(uint8_t c)
{
uint8_t line;
uint8_t ch = 0;
uint16_t i;
for(i=0;i<5;i++)
{
ch = FontLookup[c-32][i];
LCD5110_WriteByte(ch,0);
}
}
void LCD5110_Write_String(uint8_t X,uint8_t Y,char *s)
{
LCD5110_Set_XY(X,Y);
while(*s)
{
LCD5110_Write_Char(*s++);
}
}
void LCD5110_Write_Num(uint8_t X,uint8_t Y,uint32_t num)
{
char str[10];
sprintf(str,"%d",num);
LCD5110_Write_String(X,Y,str);
}
uint8_t KeyScan(void)
{
uint8_t keyvalue = 0xff;
GPIO_ResetBits(GPIOA,ROW1);
if(GPIO_ReadInputDataBit(GPIOB,COL1)==0)
keyvalue = 1;
if(GPIO_ReadInputDataBit(GPIOB,COL2)==0)
keyvalue = 2;
if(GPIO_ReadInputDataBit(GPIOB,COL3)==0)
keyvalue = 3;
if(GPIO_ReadInputDataBit(GPIOB,COL4)==0)
keyvalue = 10;
GPIO_SetBits(GPIOA,ROW1);
GPIO_ResetBits(GPIOA,ROW2);
if(GPIO_ReadInputDataBit(GPIOB,COL1)==0)
keyvalue = 4;
if(GPIO_ReadInputDataBit(GPIOB,COL2)==0)
keyvalue = 5;
if(GPIO_ReadInputDataBit(GPIOB,COL3)==0)
keyvalue = 6;
if(GPIO_ReadInputDataBit(GPIOB,COL4)==0)
keyvalue = 11;
GPIO_SetBits(GPIOA,ROW2);
GPIO_ResetBits(GPIOA,ROW3);
if(GPIO_ReadInputDataBit(GPIOB,COL1)==0)
keyvalue = 7;
if(GPIO_ReadInputDataBit(GPIOB,COL2)==0)
keyvalue = 8;
if(GPIO_ReadInputDataBit(GPIOB,COL3)==0)
keyvalue = 9;
if(GPIO_ReadInputDataBit(GPIOB,COL4)==0)
keyvalue = 12;
GPIO_SetBits(GPIOA,ROW3);
GPIO_ResetBits(GPIOA,ROW4);
if(GPIO_ReadInputDataBit(GPIOB,COL1)==0)
keyvalue = 14;
if(GPIO_ReadInputDataBit(GPIOB,COL2)==0)
keyvalue = 0;
if(GPIO_ReadInputDataBit(GPIOB,COL3)==0)
keyvalue = 15;
if(GPIO_ReadInputDataBit(GPIOB,COL4)==0)
keyvalue = 13;
GPIO_SetBits(GPIOA,ROW4);
return keyvalue;
}
int main(void)
{
uint8_t keyvalue = 0xff;
uint8_t num1 = 0,num2 = 0;
uint8_t op = 0;
uint32_t result = 0;
char str[10];
GPIO_Configuration();
LCD5110_GPIO_Configuration();
LCD5110_Init();
LCD5110_Clear();
while(1)
{
keyvalue = KeyScan();
if(keyvalue!=0xff)
{
if(keyvalue>=0&&keyvalue<=9)
{
if(op==0)
{
num1 = num1*10+keyvalue;
sprintf(str,"%d",num1);
LCD5110_Write_String(0,0,str);
}
else
{
num2 = num2*10+keyvalue;
sprintf(str,"%d",num2);
LCD5110_Write_String(0,1,str);
}
}
else if(keyvalue>=10&&keyvalue<=13)
{
op = keyvalue;
LCD5110_Write_Char(op+47);
}
else if(keyvalue==14)
{
num1 = 0;
num2 = 0;
op = 0;
result = 0;
LCD5110_Clear();
}
else if(keyvalue==15)
{
switch(op)
{
case 10:
result = num1+num2;
break;
case 11:
result = num1-num2;
break;
case 12:
result = num1*num2;
break;
case 13:
result = num1/num2;
break;
default:
break;
}
sprintf(str,"%d",result);
LCD5110_Write_String(0,2,str);
}
}
}
}
```
阅读全文
相关推荐
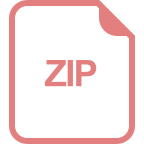
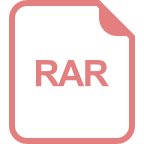
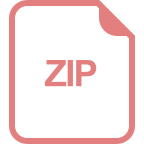

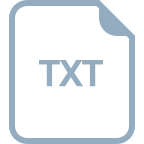




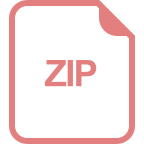
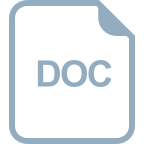
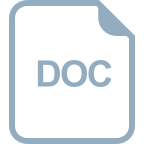

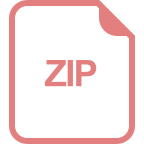
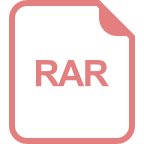
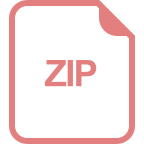
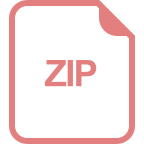