C#使用远程主机上的bat文件启动软件
时间: 2024-10-24 18:13:00 浏览: 35
在C#中,你可以通过创建进程或者使用Powershell来启动远程主机上的批处理文件(.bat)。这里我们使用Process.Start()方法来启动远程计算机上的应用程序,假设批处理文件是用来运行某个软件的安装程序或执行其他操作。
首先,你需要获取到远程计算机的IP地址、用户名和密码以进行身份验证。然后,可以利用如下的代码片段:
```csharp
using System.Diagnostics;
using System.Net.NetworkInformation;
using System.Security.Principal;
using System.Text;
public static void LaunchBatchFileRemotely(string remoteMachine, string batFilePath)
{
NetworkCredential creds = new NetworkCredential("your_username", "your_password"); // 替换为实际的用户名和密码
// 获取远程计算机的IP地址
IPHostEntry host = Dns.GetHostEntry(remoteMachine);
if(host.AddressList.Count > 0)
{
string ipAddress = host.AddressList[0].ToString(); // 假设只有一个IP地址
ProcessStartInfo psi = new ProcessStartInfo();
psi.UseShellExecute = true; // 启动远程进程时使用默认的shell(批处理)
psi.WorkingDirectory = @"\path\to\dir\on\remote\computer"; // 设置批处理文件的工作目录
psi.FileName = @"\\{ipAddress}\{batFilePath}"; // 文件路径,注意网络路径格式
psi.Username = creds.UserName;
psi.Password = creds.Password;
try
{
// 在本地运行一个命令,这个命令会尝试在远程主机上执行指定的批处理文件
Process.Start(psi);
}
catch (Exception ex)
{
Console.WriteLine($"Error launching batch file remotely: {ex.Message}");
}
}
else
{
Console.WriteLine($"Failed to get IP address for {remoteMachine}. Please check the input.");
}
}
// 调用方法,例如:
LaunchBatchFileRemotely("remote-machine", "install.bat");
```
请注意,这只是一个基本示例,实际应用中可能需要处理更多的异常情况,并确保有足够的权限访问远程主机。
阅读全文
相关推荐

















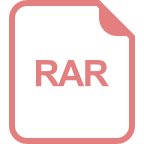