springbootmail邮件正文内容发送html 含img
时间: 2023-11-05 22:03:30 浏览: 84
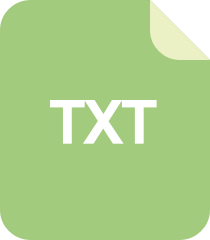
用Java Mail发送带有图片的html格式的邮件.txt

使用Spring Boot发送包含图像的HTML邮件很简单。下面是一个使用Spring Boot Mail的示例代码:
首先,在pom.xml文件中添加以下依赖:
```xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
</dependencies>
```
然后,在application.properties文件中配置邮件服务器相关的属性:
```properties
spring.mail.host=your-mail-server-host
spring.mail.port=your-mail-server-port
spring.mail.username=your-mail-username
spring.mail.password=your-mail-password // 如果是SMTP授权码,需要使用授权码而不是邮箱密码
spring.mail.properties.mail.smtp.auth=true
spring.mail.properties.mail.smtp.starttls.enable=true
spring.mail.properties.mail.smtp.starttls.required=true
```
接下来,创建一个EmailService类来发送邮件:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.mail.javamail.JavaMailSender;
import org.springframework.mail.javamail.MimeMessageHelper;
import org.springframework.stereotype.Service;
import javax.mail.MessagingException;
import javax.mail.internet.MimeMessage;
import java.io.File;
import java.util.Map;
@Service
public class EmailService {
@Autowired
private JavaMailSender javaMailSender;
public void sendEmailWithImage(String to, String subject, String htmlContent, Map<String, File> images) throws MessagingException {
MimeMessage message = javaMailSender.createMimeMessage();
MimeMessageHelper helper = new MimeMessageHelper(message, true);
// 设置收件人、主题和HTML正文
helper.setTo(to);
helper.setSubject(subject);
helper.setText(htmlContent, true);
// 添加图像
for (Map.Entry<String, File> entry : images.entrySet()) {
String contentId = entry.getKey();
File image = entry.getValue();
helper.addInline(contentId, image);
}
// 发送邮件
javaMailSender.send(message);
}
}
```
最后,在你的业务逻辑中使用EmailService发送邮件:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import javax.mail.MessagingException;
import java.io.File;
import java.util.HashMap;
import java.util.Map;
@RestController
public class EmailController {
@Autowired
private EmailService emailService;
@GetMapping("/send")
public String sendEmail() {
try {
// 邮件收件人
String to = "recipient@example.com";
// 邮件主题
String subject = "测试邮件";
// 邮件内容 HTML
String htmlContent = "<h1>欢迎使用Spring Boot Mail</h1><p>这是一封包含图像的HTML邮件。</p><p><img src='cid:image1'></p>";
// 图像文件路径
String imagePath = "/path/to/your/image.jpg";
File imageFile = new File(imagePath);
// 将图像添加到Map中
Map<String, File> images = new HashMap<>();
images.put("image1", imageFile);
// 发送邮件
emailService.sendEmailWithImage(to, subject, htmlContent, images);
return "邮件发送成功";
} catch (MessagingException e) {
e.printStackTrace();
return "邮件发送失败";
}
}
}
```
这样,当你调用`/send`接口时,邮件将被发送到指定收件人,邮件正文将包含一个图像。确保替换`to`、`subject`和`imagePath`变量以及邮件服务器的配置信息。
希望这可以帮助到你!
阅读全文
相关推荐
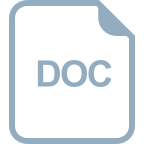
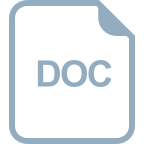
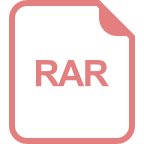
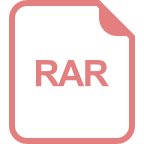
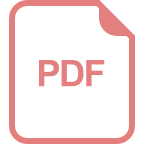
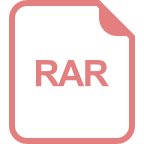
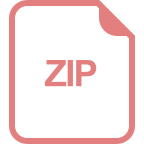
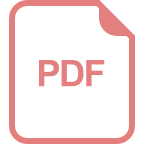
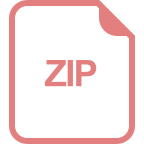
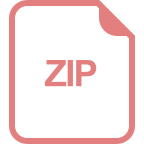
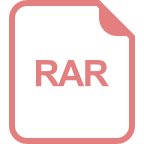
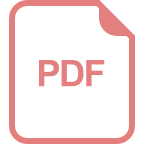
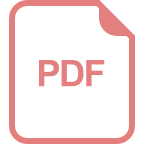
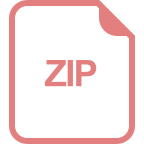
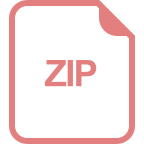