springboot实现上传文件下载文件
时间: 2023-08-17 22:09:13 浏览: 103
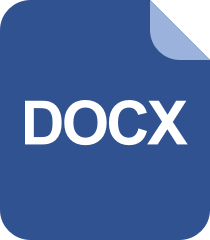
SpringBoot实现文件上传和下载.docx
Spring Boot 提供了方便的方式来实现文件上传和下载功能。下面是一个简单的示例:
1. 文件上传:
首先,需要在 Spring Boot 的配置文件中配置文件上传的相关参数:
```
# application.properties
spring.servlet.multipart.max-file-size=10MB
spring.servlet.multipart.max-request-size=10MB
```
然后,创建一个控制器来处理文件上传请求:
```java
import org.springframework.util.StringUtils;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
@RestController
public class FileUploadController {
@PostMapping("/upload")
public String uploadFile(@RequestParam("file") MultipartFile file) {
// 检查文件是否为空
if (file.isEmpty()) {
return "请选择要上传的文件";
}
// 获取文件名
String fileName = StringUtils.cleanPath(file.getOriginalFilename());
try {
// 保存文件到指定路径
// 这里可以根据需求自定义保存路径
// 例如:String uploadDir = "C:/uploads/";
// String filePath = uploadDir + fileName;
// file.transferTo(new File(filePath));
// 返回成功信息
return "文件上传成功";
} catch (IOException e) {
e.printStackTrace();
return "文件上传失败";
}
}
}
```
2. 文件下载:
创建一个控制器来处理文件下载请求:
```java
import org.springframework.core.io.Resource;
import org.springframework.core.io.UrlResource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
import java.io.IOException;
import java.net.MalformedURLException;
import java.nio.file.Path;
import java.nio.file.Paths;
@RestController
public class FileDownloadController {
@GetMapping("/download/{fileName:.+}")
public ResponseEntity<Resource> downloadFile(@PathVariable String fileName) {
// 根据文件名获取文件路径
// 这里可以根据需求自定义文件路径
// 例如:String uploadDir = "C:/uploads/";
// String filePath = uploadDir + fileName;
// Path path = Paths.get(filePath);
// 根据路径创建 Resource 对象
Resource resource;
try {
resource = new UrlResource(path.toUri());
} catch (MalformedURLException e) {
e.printStackTrace();
return ResponseEntity.notFound().build();
}
// 设置下载响应头
HttpHeaders headers = new HttpHeaders();
headers.add(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + resource.getFilename() + "\"");
try {
// 返回文件下载响应
return ResponseEntity.ok()
.headers(headers)
.contentType(MediaType.APPLICATION_OCTET_STREAM)
.body(resource);
} catch (IOException e) {
e.printStackTrace();
return ResponseEntity.notFound().build();
}
}
}
```
以上示例演示了如何在 Spring Boot 中实现文件上传和下载功能。你可以根据实际需求进行相应的调整和扩展。
阅读全文
相关推荐
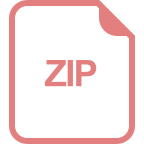
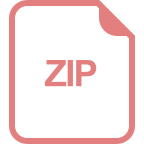









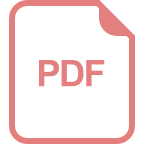
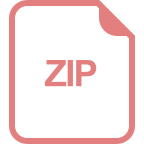
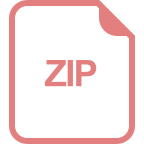
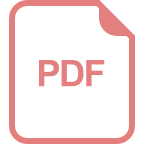
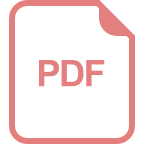
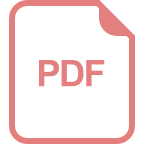
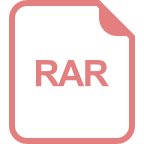