帮我用c#编写一个Adam4150控制电灯开关的代码
时间: 2024-09-24 16:15:50 浏览: 53
在C#中,我们通常会使用.NET框架中的System.IO.Ports命名空间来控制串口通信。假设你有一个名为Adam4150的设备,它通过串口连接并且支持特定的命令协议来操作电灯开关。以下是一个简单的示例,展示了如何使用System.IO.Ports.SerialPort类来发送控制信号:
```csharp
using System;
using System.IO.Ports;
class Program
{
static void Main(string[] args)
{
// 创建SerialPort实例
SerialPort port = new SerialPort("COM1", 9600, Parity.None, 8, StopBits.One); // 替换为实际的端口号
try
{
// 打开串口
port.Open();
// 检查设备是否就绪
if (port.IsOpen)
{
// 发送打开或关闭电灯的命令(例如:0x01代表打开,0x00代表关闭)
byte[] commandToSwitchOn = { 0x01 }; // 灯开
byte[] commandToSwitchOff = { 0x00 }; // 灯关
// 发送命令
port.Write(commandToSwitchOn); // 开灯
Console.WriteLine("Sent command to switch on the light.");
// 延迟一段时间以等待反应
System.Threading.Thread.Sleep(1000); // 这里可以调整时间以适应实际需要
port.Write(commandToSwitchOff); // 关灯
Console.WriteLine("Sent command to switch off the light.");
}
else
{
Console.WriteLine("Error: Unable to open serial port.");
}
}
catch (Exception ex)
{
Console.WriteLine($"An error occurred: {ex.Message}");
}
// 关闭串口
port.Close();
}
}
```
注意:
1. 你需要替换`"COM1"`为你计算机上实际的串口路径。
2. Adam4150的具体命令可能因设备而异,这里仅作示例。
3. 上述代码没有包含错误处理,实际应用中应添加更详细的异常捕获。
阅读全文
相关推荐




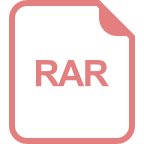
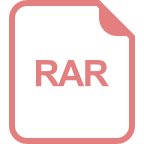
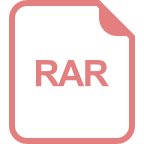
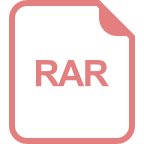

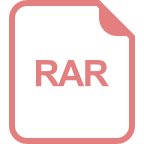
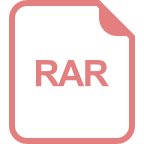
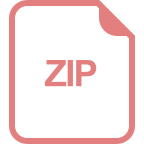




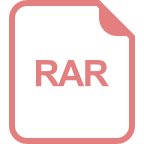