python怎么导入一个自制数据集
时间: 2024-10-16 17:11:31 浏览: 29
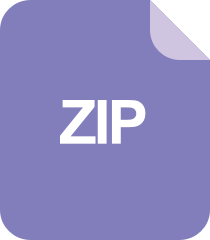
使用自制数据集实现动物种类语音识别-python毕业设计
在Python中导入自定义数据集通常包括以下几个步骤:
1. **文件存储格式**:首先确认你的数据集是以哪种格式保存的。常见的格式有CSV、Excel(`.xls` 或 `.xlsx`)、JSON、文本文件等。Python有相应的库(如pandas对CSV和Excel,json库对JSON)来进行读取。
2. **使用pandas**:如果你的数据是CSV或Excel文件,可以使用pandas库的`read_csv()`或`read_excel()`函数。例如:
```python
import pandas as pd
# 读取CSV文件
df_custom = pd.read_csv('your_dataset.csv')
# 读取Excel文件
df_custom = pd.read_excel('your_dataset.xlsx')
```
3. **使用json库**:如果数据是JSON格式,用`json.load()`或`json.loads()`:
```python
import json
with open('your_dataset.json') as f:
dataset_dict = json.load(f)
# 将字典转换成DataFrame
df_custom = pd.DataFrame(dataset_dict)
```
4. **文本文件**:如果是文本文件,每行代表一条记录,可以使用`open()`打开后逐行读取:
```python
with open('your_dataset.txt', 'r') as file:
lines = file.readlines()
records = [line.strip() for line in lines] # 分割并清理换行符
```
5. **自定义解析函数**:对于非标准格式,你可以编写自定义函数来解析数据。例如,CSV中有特定列名的情况,可以用csv模块配合`DictReader`:
```python
import csv
def parse_row(row):
return {col_name: value for col_name, value in row}
with open('your_dataset.csv', newline='') as csvfile:
reader = csv.DictReader(csvfile)
df_custom = pd.DataFrame([parse_row(row) for row in reader])
```
记住,在导入之前确保路径正确,并且数据集已经存在。
阅读全文
相关推荐
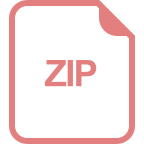
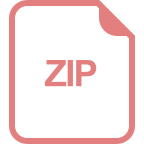

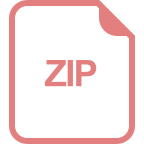
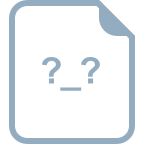
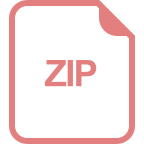
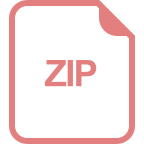
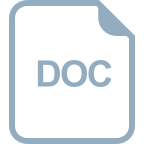
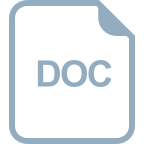
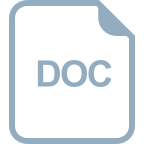
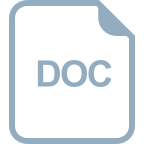







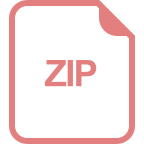