I2C外设(AT24C02)字符串读写测试例程
时间: 2024-10-15 22:06:03 浏览: 24
I2C(Inter-Integrated Circuit)是一种用于连接微控制器和其他外围设备的串行通信标准,AT24C02是一种常用的基于I2C接口的存储芯片,它主要用于非易失性的数据存储,例如字节级的数据存储。
对于AT24C02的字符串读写测试例程,通常会在嵌入式开发环境中编写,比如使用C语言配合I2C库如mraa、Wire等。这里提供一个简化的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include "mraa/i2c.h"
// AT24C02 I2C地址 (7bit slave address)
#define I2C_ADDR 0x50
void write_string(char *data) {
int len = strlen(data);
for (int i = 0; i < len; i++) {
mraa_i2c_write(I2C_ADDR, data[i], 1); // Write each character individually
}
}
char* read_string() {
char buffer[256]; // Assuming max string length is 255 characters + null terminator
memset(buffer, '\0', sizeof(buffer)); // Clear buffer
for (int i = 0; i < 255; i++) {
int byte;
if (mraa_i2c_read(I2C_ADDR, &byte, 1) == 1) {
buffer[i] = byte; // Store read byte in buffer
} else {
break; // Stop reading if no more bytes are available
}
}
buffer[255] = '\0'; // Add null terminator at the end
return buffer;
}
int main(void) {
mraa_init(); // Initialize MRAA library
int i2c_dev = mraa_i2c_init(0); // Open I2C bus
if (!i2c_dev) {
printf("Failed to initialize I2C\n");
return -1;
}
// Test write
char test_string[] = "Hello, I2C!";
printf("Writing string: %s\n", test_string);
write_string(test_string);
// Test read
char* read_str = read_string();
printf("Read string: %s\n", read_str);
mraa_i2c_close(i2c_dev); // Close I2C connection
mraa_cleanup();
return 0;
}
```
这个示例首先初始化I2C,并设置AT24C02的地址,然后分别实现了写字符串和读取字符串的功能。注意这只是一个基本示例,实际应用可能需要处理错误并可能需要更复杂的同步逻辑。
阅读全文
相关推荐
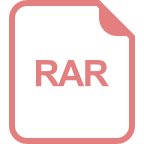
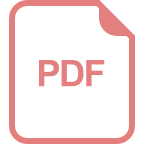
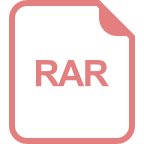
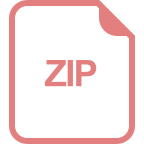
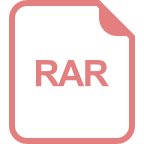
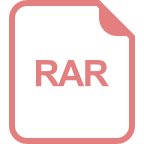
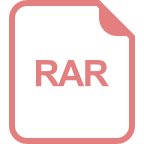
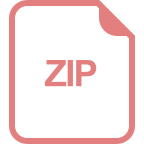
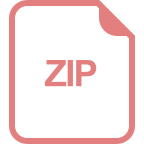
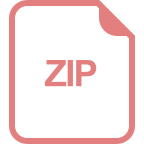
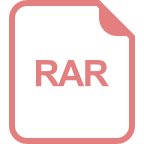
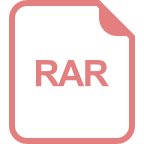
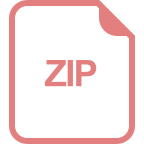
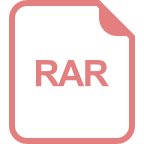
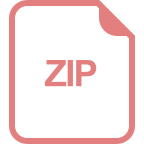
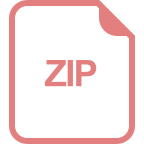