编写一个金额找零的系统,用输入框输入一个整数,表示找零的数量,数值为1- 100。假如系统中有50、20、10、5、1这5种面额的纸币,显示每种纸币应该找的数量。例如 78元应该为50元1张、20元1张、5元1张、1元3张。 jsp代码
时间: 2024-09-25 07:01:46 浏览: 65
要编写一个基于JSP的金额找零系统,首先你需要创建一个HTML表单,让用户输入找零金额,然后通过JSP页面处理这个数据并计算出每种面额的纸币数量。以下是一个简单的JSP和Java后台处理的示例:
**HTML部分 (index.jsp)**:
```html
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<title>金额找零</title>
</head>
<body>
<h2>请输入找零金额:</h2>
<form action="findChange.jsp" method="post">
<input type="number" id="totalAmount" name="totalAmount" min="1" max="100" required>
<input type="submit" value="计算找零">
</form>
</body>
</html>
```
**Java后台 (FindChangeServlet.java)** (假设在`WEB-INF`目录下的`servlets`包下):
```java
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.*;
public class FindChangeServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
int totalAmount = Integer.parseInt(request.getParameter("totalAmount"));
int[] change = {50, 20, 10, 5, 1}; // 面额数组
// 计算每种面额的找零数量
int[] changeCount = calculateChange(totalAmount, change);
// 将结果渲染到JSP页面
request.setAttribute("change", changeCount);
RequestDispatcher dispatcher = getServletContext()..getRequestDispatcher("showChange.jsp");
dispatcher.forward(request, response);
}
private int[] calculateChange(int total, int[] denominations) {
int[] result = new int[denominations.length];
for (int i = 0; i < denominations.length; i++) {
while (total >= denominations[i]) {
result[i]++;
total -= denominations[i];
}
}
return result;
}
}
```
**JSP部分 (showChange.jsp):**
```jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>找零结果</title>
</head>
<body>
<h2>找零结果:</h2>
<table border="1">
<tr>
<th>面额</th>
<th>数量</th>
</tr>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<c:forEach items="${change}" var="coin" index="index">
<tr>
<td>${coin}</td>
<td><c:out value="${coin}" /></td> <!-- 输出纸币数量 -->
</tr>
</c:forEach>
</table>
</body>
</html>
```
在这个例子中,用户在`index.jsp`中输入找零金额后,提交到`FindChangeServlet`,该Servlet会计算出每种面额的纸币数量,并将结果传递给`showChange.jsp`页面显示。
阅读全文
相关推荐
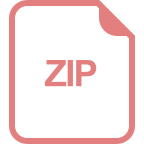
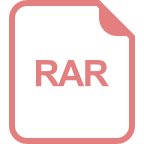
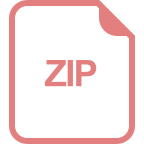





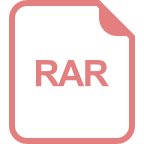
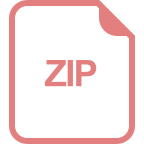
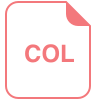

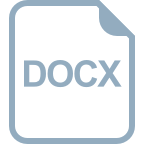
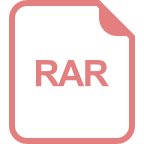