List<FlowVariablePo> objects = new ArrayList<>(); lambda表达式遍历元素处理元素,然后返回一个list
时间: 2024-12-26 17:29:27 浏览: 3
你可以使用Java 8的lambda表达式来遍历`List<FlowVariablePo>`并处理每个元素,最后创建一个新的List。这里是一个示例:
```java
List<FlowVariablePo> objects = new ArrayList<>(); // 假设objects已填充了一些FlowVariablePo实例
// 使用lambda表达式遍历并处理元素(假设我们只想取每个FlowVariablePo的id)
List<Long> ids = objects.stream()
.map(FlowVariablePo::getId) // 获取每个FlowVariablePo的id
.collect(Collectors.toList()); // 收集到新的List中
// 如果你想要去除重复的ids
List<Long> distinctIds = ids.stream()
.distinct() // 去除重复
.collect(Collectors.toList());
```
在这个例子中,`map`函数应用了一个函数接口(`Function<FlowVariablePo, Long>`)到列表的每个元素上,`getId()`方法代表对每个`FlowVariablePo`对象调用其`getId`方法。`collect`方法则用于收集结果。
相关问题
public static String setTypeAliasesPackage(String typeAliasesPackage) { ResourcePatternResolver resolver = new PathMatchingResourcePatternResolver(); MetadataReaderFactory metadataReaderFactory = new CachingMetadataReaderFactory(resolver); List<String> allResult = new ArrayList<>(); try { for (String aliasesPackage : typeAliasesPackage.split(",")) { List<String> result = new ArrayList<>(); aliasesPackage = ResourcePatternResolver.CLASSPATH_ALL_URL_PREFIX + ClassUtils.convertClassNameToResourcePath(aliasesPackage.trim()) + "/" + DEFAULT_RESOURCE_PATTERN; Resource[] resources = resolver.getResources(aliasesPackage); if (resources != null && resources.length > 0) { MetadataReader metadataReader = null; for (Resource resource : resources) { if (resource.isReadable()) { metadataReader = metadataReaderFactory.getMetadataReader(resource); try { result.add(Class.forName(metadataReader.getClassMetadata().getClassName()).getPackage().getName()); } catch (ClassNotFoundException e) { log.debug(e.getMessage()); } } } } if (!result.isEmpty()) { HashSet<String> hashResult = new HashSet<>(result); allResult.addAll(hashResult); } } if (!allResult.isEmpty()) { typeAliasesPackage = String.join(",", (String[]) allResult.toArray(new String[0])); } else { throw new ServiceException("mybatis typeAliasesPackage 路径扫描错误,参数typeAliasesPackage:" + typeAliasesPackage + "未找到任何包"); } } catch (IOException e) { log.debug(e.getMessage()); } return typeAliasesPackage; } 优化这段代码
首先,这段代码的作用是从给定的包路径中获取所有的类并返回这些类所在的包路径,用于配置 MyBatis 的类型别名。
接下来,我列出了一些可以优化的地方:
1. 对于每个包路径,都会进行一次 ResourcePatternResolver 的初始化。可以将其提到方法外部,避免重复创建。
2. 使用了两层循环对每个包路径中的类进行遍历,效率较低。可以将其改为单层循环,并使用 Lambda 表达式优化代码。
3. 在获取所有结果后,使用了 HashSet 进行去重。可以在遍历时使用 Set 存储结果,避免重复添加。
综上所述,下面是我优化后的代码:
```
public static String setTypeAliasesPackage(String typeAliasesPackage) {
ResourcePatternResolver resolver = new PathMatchingResourcePatternResolver();
MetadataReaderFactory metadataReaderFactory = new CachingMetadataReaderFactory(resolver);
List<String> allResult = new ArrayList<>();
String[] packages = typeAliasesPackage.split(",");
try {
for (String pkg : packages) {
String pattern = ResourcePatternResolver.CLASSPATH_ALL_URL_PREFIX + ClassUtils.convertClassNameToResourcePath(pkg.trim()) + "/" + DEFAULT_RESOURCE_PATTERN;
Resource[] resources = resolver.getResources(pattern);
Set<String> result = Arrays.stream(resources)
.filter(Resource::isReadable)
.map(resource -> {
try {
MetadataReader metadataReader = metadataReaderFactory.getMetadataReader(resource);
return Class.forName(metadataReader.getClassMetadata().getClassName()).getPackage().getName();
} catch (ClassNotFoundException | IOException e) {
log.debug(e.getMessage());
return null;
}
})
.filter(Objects::nonNull)
.collect(Collectors.toSet());
if (!result.isEmpty()) {
allResult.addAll(result);
} else {
throw new ServiceException("mybatis typeAliasesPackage 路径扫描错误,参数typeAliasesPackage:" + pkg + "未找到任何包");
}
}
return String.join(",", allResult);
} catch (IOException e) {
log.debug(e.getMessage());
return typeAliasesPackage;
}
}
```
优化这段代码:List<CompletableFuture<ContactsIntersectionVo>> futureList = intersectionResult.entrySet().stream().map(entry -> CompletableFuture.supplyAsync(() -> { String account = entry.getKey(); List<String> personNoList = entry.getValue().stream().distinct().collect(Collectors.toList()); if (personNoList.size() >= 2) {// 取两个以上的交集 List<Map<String, Object>> remarkList = Lists.newArrayList(); List<PersonBasicVo> personVoList = Lists.newArrayList(); // 获取备注、涉案人 for (String personNo : personNoList) { Map<String, Object> contactsMap = contactsMapList.stream().filter(map -> personNo.equals(map.get("personNo"))).findAny().get(); List<ContactsBasic> contactsList = (List<ContactsBasic>) contactsMap.get("contactsList"); // 获取备注 for (ContactsBasic contacts : contactsList) { if (account.equals(contacts.getRelationshipAccount())) { PersonBasicVo personBasic = personList.stream().filter(person -> personNo.equals(person.getPersonNo())).findAny().get(); Map<String, Object> remarkMap = new HashMap<>(); remarkMap.put("name", personBasic.getName()); remarkMap.put("remark", contacts.getRelationshipName()); remarkList.add(remarkMap); break; } } // 获取涉案人 personVoList.add(personList.stream().filter(person -> personNo.equals(person.getPersonNo())).findAny().get()); } // 共同号码是否属于涉案人 String commonPersonName = getCommonPersonName(personList, account); ContactsIntersectionVo contactsVo = new ContactsIntersectionVo(); contactsVo.setRemarks(remarkList); contactsVo.setPersons(personVoList); contactsVo.setCommonAccount(account); contactsVo.setCommonPersonName(commonPersonName); return contactsVo; } else { return null; } }, executor)).collect(Collectors.toList()); contactisVoList.addAll(futureList.stream().map(CompletableFuture::join) .filter(Objects::nonNull) .collect(Collectors.toList()));
优化后的代码如下:
```java
List<CompletableFuture<ContactsIntersectionVo>> futureList = intersectionResult.entrySet().stream()
.map(entry -> CompletableFuture.supplyAsync(() -> {
String account = entry.getKey();
List<String> personNoList = entry.getValue().stream().distinct().collect(Collectors.toList());
if (personNoList.size() >= 2) {// 取两个以上的交集
List<Map<String, Object>> remarkList = personNoList.stream()
.flatMap(personNo -> {
Map<String, Object> contactsMap = contactsMapList.stream()
.filter(map -> personNo.equals(map.get("personNo")))
.findAny()
.orElseThrow(() -> new NoSuchElementException("Person not found"));
List<ContactsBasic> contactsList = (List<ContactsBasic>) contactsMap.get("contactsList");
PersonBasicVo personBasic = personList.stream()
.filter(person -> personNo.equals(person.getPersonNo()))
.findAny()
.orElseThrow(() -> new NoSuchElementException("Person not found"));
return contactsList.stream()
.filter(contacts -> account.equals(contacts.getRelationshipAccount()))
.flatMap(contacts -> Stream.of(Map.of("name", personBasic.getName(), "remark", contacts.getRelationshipName())));
})
.collect(Collectors.toList());
List<PersonBasicVo> personVoList = personNoList.stream()
.map(personNo -> personList.stream()
.filter(person -> personNo.equals(person.getPersonNo()))
.findAny()
.orElseThrow(() -> new NoSuchElementException("Person not found")))
.collect(Collectors.toList());
String commonPersonName = getCommonPersonName(personList, account);
ContactsIntersectionVo contactsVo = new ContactsIntersectionVo();
contactsVo.setRemarks(remarkList);
contactsVo.setPersons(personVoList);
contactsVo.setCommonAccount(account);
contactsVo.setCommonPersonName(commonPersonName);
return contactsVo;
} else {
return null;
}
}, executor))
.collect(Collectors.toList());
contactisVoList.addAll(futureList.stream()
.map(CompletableFuture::join)
.filter(Objects::nonNull)
.collect(Collectors.toList()));
```
主要优化:
1. 使用流式编程,替换原来的for循环和entrySet遍历。
2. 使用`stream()`和`collect(Collectors.toList())`方法来收集结果,代替手动添加到列表中。
3. 使用`flatMap`方法来处理嵌套的列表。
4. 使用`orElseThrow`方法来处理可能找不到元素的情况,避免空指针异常。
5. 将lambda表达式内联,使代码更简洁。
阅读全文
相关推荐
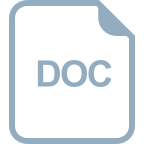
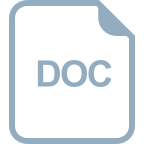
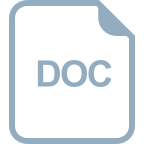
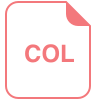
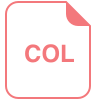
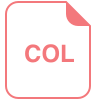
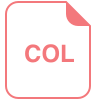
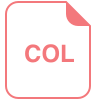
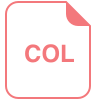
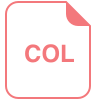
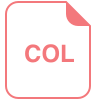
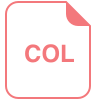
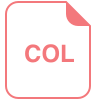
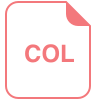


