运用结构指针数组进行插入排序
时间: 2024-06-16 09:00:47 浏览: 11
结构指针数组是由结构体类型的指针组成的数组。在进行插入排序时,可以通过结构指针数组的方式来对结构体数组进行排序。
具体的插入排序实现方法如下:
1. 定义一个结构体类型,包含需要排序的元素。
2. 定义一个结构体指针数组,数组元素为指向结构体类型的指针。
3. 通过循环将需要排序的元素存储到结构体数组中。
4. 对结构体指针数组进行插入排序,将每个元素依次插入到已排序的部分中。
5. 循环输出排序后的结果。
以下是一个示例代码,用于演示如何使用结构指针数组进行插入排序:
```
#include <stdio.h>
#include <stdlib.h>
struct student {
char name[20];
int score;
};
void insertion_sort(struct student** array, int length) {
int i, j;
struct student* temp;
for (i = 1; i < length; i++) {
temp = array[i];
for (j = i - 1; j >= 0 && array[j]->score > temp->score; j--) {
array[j + 1] = array[j];
}
array[j + 1] = temp;
}
}
int main() {
int i, n;
struct student* array[100];
printf("请输入学生人数:");
scanf("%d", &n);
for (i = 0; i < n; i++) {
array[i] = (struct student*)malloc(sizeof(struct student));
printf("请输入第%d个学生的姓名和分数:", i + 1);
scanf("%s %d", array[i]->name, &array[i]->score);
}
insertion_sort(array, n);
printf("排序后的结果为:\n");
for (i = 0; i < n; i++) {
printf("%s %d\n", array[i]->name, array[i]->score);
free(array[i]);
}
return 0;
}
相关推荐
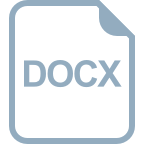
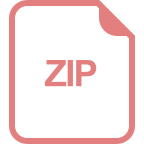
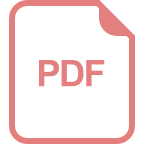















