在c语言中,将数组int a[4][5]={{3,6,2,5,-20},{1,7,9,8,-12},{-1,-3,10,11,22},{-5,32,14,23,44}}从小到大进行排序
时间: 2024-11-27 12:04:02 浏览: 12
在 C 语言中,对二维数组进行排序通常需要先将其转换成一维数组,然后利用标准库提供的函数如 `qsort` 进行排序,最后再把结果复制回二维数组。这里是一个示例,首先假设我们有一个临时的一维整型数组 temp,用于存储二维数组的内容,然后再进行降序排列:
```c
#include <stdio.h>
#include <stdlib.h>
// 数组元素类型和大小
typedef int Type;
#define ROWS 4
#define COLS 5
void swap(Type* a, Type* b) {
Type t = *a;
*a = *b;
*b = t;
}
void print_array(Type array[ROWS][COLS], int rows, int cols) {
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
printf("%d ", array[i][j]);
}
printf("\n");
}
}
void sort_2d_array(int arr[ROWS][COLS]) {
// 将二维数组转换为一维
Type temp[ROWS * COLS];
int k = 0;
for (int i = 0; i < ROWS; ++i) {
for (int j = 0; j < COLS; ++j) {
temp[k++] = arr[i][j];
}
}
// 对一维数组进行降序排序
qsort(temp, ROWS * COLS, sizeof(Type), compare);
// 将排序后的数据复制回二维数组
k = 0;
for (int i = 0; i < ROWS; ++i) {
for (int j = 0; j < COLS; ++j) {
arr[i][j] = temp[k++];
}
}
}
// 自定义比较函数(这里是降序)
int compare(const void* a, const void* b) {
return (*(const Type*)b - *(const Type*)a);
}
int main() {
int a[ROWS][COLS] = {{3, 6, 2, 5, -20}, {1, 7, 9, 8, -12}, {-1, -3, 10, 11, 22}, {-5, 32, 14, 23, 44}};
sort_2d_array(a);
printf("Sorted 2D Array:\n");
print_array(a, ROWS, COLS);
return 0;
}
```
请注意,上述代码中的 `compare` 函数是一个自定义的降序比较函数,如果你想要升序排列,只需修改该函数即可。
阅读全文
相关推荐
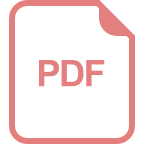
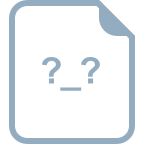
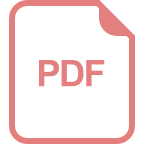
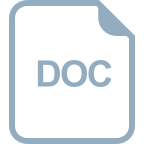
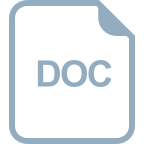
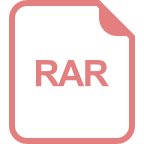
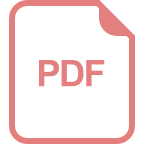
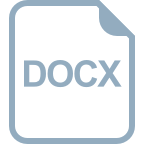
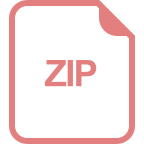
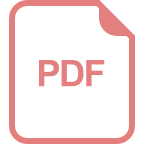
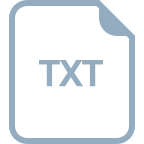
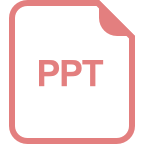
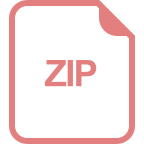
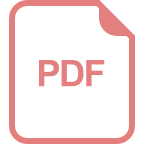
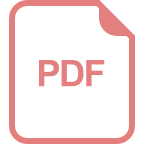
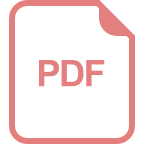
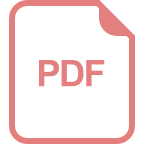