C语言输入一系列,整数统计出正整数和负整数个数输入0结束
时间: 2024-11-13 17:41:56 浏览: 29
在C语言中,你可以使用循环结构和条件判断来统计正整数、负整数和0的数量。以下是一个简单的示例程序:
```c
#include <stdio.h>
int main() {
int num, count_pos = 0, count_neg = 0;
printf("请输入一系列整数(输入0表示结束):\n");
while (scanf("%d", &num) == 1) { // 使用scanf读取整数
if (num > 0) {
count_pos++;
} else if (num < 0) {
count_neg++;
}
// 清理可能存在的换行符
getchar();
}
printf("正整数有 %d 个,负整数有 %d 个。\n", count_pos, count_neg);
return 0;
}
```
在这个程序中,用户可以逐个输入整数,每输入一个数,就检查其是否大于0(正整数),小于0(负整数)。当输入0时,`scanf`函数返回0,循环会终止。最后,显示正数和负数的计数值。
相关问题
读入一系列整数,统计出正整数的个数和负整数的个数,读入0则结束。C语言
在C语言中,你可以使用while循环和条件判断来实现这个功能。这里是一个简单的示例程序:
```c
#include <stdio.h>
int main() {
int num, count_positive = 0, count_negative = 0;
printf("请输入整数(输入0结束): ");
while (scanf("%d", &num) == 1) { // 保证每次读取的是整数
if (num > 0) {
count_positive++;
} else if (num < 0) {
count_negative++;
}
// 避免用户直接输入换行符导致死循环
if (num != 0 && getchar() != '\n') {
printf("请输入整数: ");
}
}
printf("正整数有 %d 个,负整数有 %d 个。\n", count_positive, count_negative);
return 0;
}
```
在这个程序里,我们使用`scanf()`函数从用户那里读取整数,然后通过`if`语句检查数值的正负并计数。当遇到0或者用户输入了非数字字符(如空格、换行符等),我们就跳出循环。
c语言2.统计正整数、负整数和0的个数 ([问题描述]输入若干个整数,以-32767作为结束标志,分别统计正整数、负整数和的个数,结束标记不算在内。[输入形式]若干个整数。 [输出形式]正整数、负整数和的个数。
在C语言中,你可以使用循环结构如for或while,以及一个数组来跟踪每个类型的计数。这里是一个简单的示例程序:
```c
#include <stdio.h>
int main() {
int num, positive_count = 0, negative_count = 0;
int zero_count = 0;
while (scanf("%d", &num) == 1 && num != -32767) { // 读取整数直到遇到-32767
if (num > 0) {
positive_count++;
} else if (num < 0) {
negative_count++;
} else {
zero_count++;
}
}
printf("正整数个数: %d\n", positive_count);
printf("负整数个数: %d\n", negative_count);
printf("零的个数: %d\n", zero_count);
return 0;
}
```
这个程序首先初始化了三个计数器:positive_count(用于正整数)、negative_count(用于负整数)和zero_count(用于零)。然后在一个无限循环中,它读取用户输入的一个整数。如果数大于零,增加positive_count;小于零,增加negative_count;等于零,增加zero_count。当输入的数等于-32767时,跳出循环,并打印出每种类型的计数值。
阅读全文
相关推荐
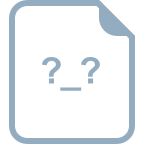
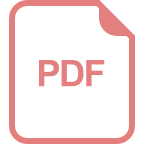













