Unity中实现物体弯曲
时间: 2024-04-17 11:28:35 浏览: 382
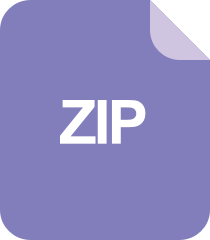
Obi Rope 3.2.zip
在Unity中实现物体弯曲有多种方法,以下是其中一种常见的方法:
1. 创建一个空物体作为弯曲的曲线路径。可以使用Bezier曲线、Catmull-Rom样条曲线或自定义路径来定义弯曲形状。
2. 创建要弯曲的物体,例如一个平面或立方体。
3. 将物体放置在曲线路径上,可以使用曲线上的点进行定位。
4. 使用曲线路径上的点来计算物体的新位置和旋转角度。可以使用插值方法(如Lerp或Slerp)来平滑地移动物体沿着曲线路径。
5. 在每一帧更新物体的位置和旋转角度,使其跟随曲线路径弯曲。
以下是一个简单的示例代码,用于在Unity中实现物体沿着曲线路径弯曲:
```csharp
public class ObjectBend : MonoBehaviour
{
public Transform curvePath; // 曲线路径
public float speed = 1f; // 移动速度
private float currentT = 0f; // 当前位置参数(0到1之间)
void Update()
{
currentT += speed * Time.deltaTime; // 更新位置参数
if (currentT > 1f) // 到达终点后重置位置参数
{
currentT = 0f;
}
Vector3 position = curvePath.position; // 获取路径起始点位置
Quaternion rotation = curvePath.rotation; // 获取路径起始点旋转角度
// 根据当前位置参数在路径上进行插值计算
position = Vector3.Lerp(curvePath.position, curvePath.GetChild(1).position, currentT);
rotation = Quaternion.Slerp(curvePath.rotation, curvePath.GetChild(1).rotation, currentT);
transform.position = position; // 更新物体位置
transform.rotation = rotation; // 更新物体旋转角度
}
}
```
在上面的示例中,我们使用了一个曲线路径对象来定义物体的弯曲形状。物体通过插值计算在路径上移动,并根据路径上的点进行位置和旋转的插值计算,以实现物体的弯曲效果。请注意,这只是一个简单示例,实际应用中可能需要更复杂的逻辑和算法来实现所需的效果。
阅读全文
相关推荐
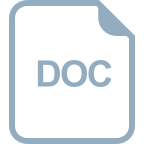
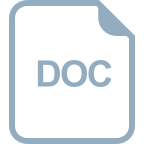
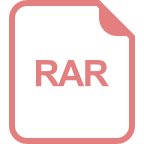
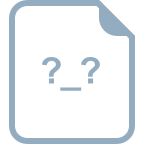
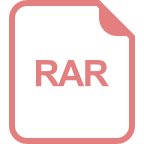
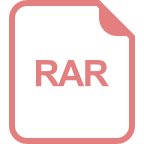
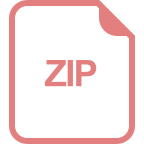
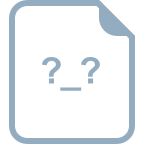
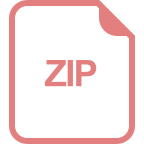
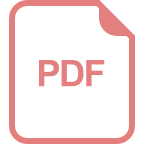
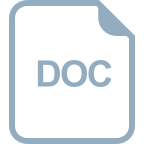





