假设每班人数最多不超过30人,具体人数由键盘输入, 试编程打印最高分及其学号。 要求:用一维数组和指针变量作函数参数,编程打印某班一门课成绩的最高分和学号。请编写相应的程序代码,并调试运行出正确结果。 程序代码:
时间: 2024-12-16 15:18:25 浏览: 9
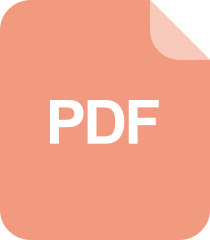
java课堂作业:用二维数组存储五个学生姓名学号,并用正则表达式判断输入数据是否有误。输出按学号排序的学生。

下面是一个简单的C++程序,它使用一维数组和指针作为函数参数,实现了计算并打印班级成绩中的最高分和对应学号的功能:
```cpp
#include <iostream>
using namespace std;
// 定义结构体表示学生信息
struct Student {
int score;
int student_id; // 学号
};
// 函数声明,接收数组、大小和指向当前元素的指针作为参数
void printTopScore(int* scores, int size, int* current) {
if (size > 0) {
*current = *current == 0 ? scores[0] : max(*current, scores[0]);
printTopScore(scores + 1, size - 1, current);
} else {
cout << "Highest score: " << *current << ", Student ID: " << (*current + 1) << endl;
}
}
int main() {
int classSize;
cout << "Enter the maximum number of students per class (<=30): ";
cin >> classSize;
if (classSize <= 0 || classSize > 30) {
cout << "Invalid class size! Please enter a number between 1 and 30." << endl;
return 0;
}
int numStudents[classSize];
for (int i = 0; i < classSize; i++) {
cout << "Enter score for student " << (i + 1) << ": ";
cin >> numStudents[i].score;
numStudents[i].student_id = i + 1; // 设置默认学号从1开始
}
// 初始化最高分和其对应的学号为第一个学生的分数
int topScore = numStudents[0].score;
int topStudentId = numStudents[0].student_id;
printTopScore(numStudents, classSize, &topScore);
return 0;
}
```
阅读全文
相关推荐
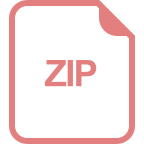
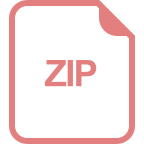















