debug spring boot
时间: 2023-10-09 19:13:29 浏览: 46
远程调试 Spring Boot 可以通过以下步骤实现:
1. 确保你的 Spring Boot 应用程序已经使用了适当的调试配置。在你的应用程序的运行环境中,打开 `application.properties` 文件,并添加以下配置:
```
spring.devtools.remote.secret=your-secret-key
spring.devtools.remote.debug.enabled=true
```
将 `your-secret-key` 替换为你自己设定的秘钥。
2. 在你的 IDE 中打开你的 Spring Boot 项目。进入 Debug 配置,添加一个新的远程调试配置。
3. 设置 Remote 调试的主机和端口。通常,默认情况下 Spring Boot 使用 8000 端口进行远程调试,你可以在启动项目时添加 `-agentlib:jdwp=transport=dt_socket,server=y,suspend=n,address=8000` 参数来指定端口。
4. 启动你的 Spring Boot 应用程序,确保它在远程调试模式下运行。
5. 在 IDE 中启动你的 Debug 配置,连接到远程应用程序。
现在你就可以在 IDE 中远程调试 Spring Boot 应用程序了。你可以设置断点、观察变量的值,并逐步执行代码来进行调试。
相关问题
debug spring boot 3 aot
AOT (Ahead-Of-Time) compilation is a way to compile code before it is executed, which can lead to faster startup times and reduced memory usage. To debug a Spring Boot 3 application with AOT compilation, you can follow these steps:
1. Add the following dependencies to your `pom.xml` file:
```xml
<dependency>
<groupId>org.springframework.experimental</groupId>
<artifactId>spring-graalvm-native</artifactId>
<version>0.9.2</version>
<scope>development</scope>
</dependency>
<dependency>
<groupId>org.springframework.experimental</groupId>
<artifactId>spring-aot</artifactId>
<version>0.9.2</version>
<scope>development</scope>
</dependency>
```
2. Build the project using Maven:
```bash
mvn clean package -Pnative
```
This will generate a native executable that can be run on your system.
3. To debug the native executable, you can use a tool like `gdb`. First, start the executable with the `gdb` command:
```bash
gdb target/application
```
4. Set a breakpoint at a specific line of code by typing:
```bash
break filename.c:line_number
```
5. Start the program by typing:
```bash
run
```
6. The program will stop at the breakpoint and you can use the `next` command to step through the code.
Note that debugging AOT-compiled code can be more difficult than debugging traditional compiled code, so it may take some experimentation to get it working properly.
spring boot 断点调试
在使用Spring Boot进行断点调试时,你可以按照以下步骤进行操作:
1. 打开你的Spring Boot项目,并确保你已经安装了适当的调试器,比如Eclipse、IntelliJ IDEA等。
2. 找到你要进行断点调试的代码位置,在该位置上设置断点。你可以在方法的起始位置、某个关键行或者条件语句上设置断点。
3. 启动你的Spring Boot项目,并等待它完全加载完成。
4. 运行调试器,连接到正在运行的Spring Boot应用程序。在Eclipse中,你可以通过点击菜单栏的"Debug"按钮,然后选择你的Spring Boot应用程序来启动调试会话。在IntelliJ IDEA中,你可以使用"Debug"按钮或者快捷键来启动调试会话。
5. 当应用程序开始运行时,它会在你设置断点的地方停下来。你可以使用调试器提供的各种功能来观察变量、查看堆栈跟踪、单步执行代码等。
6. 按需进行调试操作,比如逐行执行、观察变量值的变化、检查方法返回值等。
7. 如果需要,你还可以在断点处设置条件来控制何时触发断点,以及使用调试器提供的其他高级功能。
注意:在进行断点调试时,请确保你的代码和断点位置是正确的,并且你的调试器已经正确地配置和连接到你的Spring Boot应用程序。
相关推荐
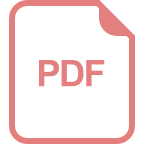
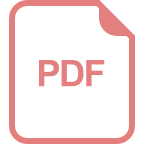












