如何在Vue 3中正确地封装和管理HTTP接口?
时间: 2024-10-17 12:09:30 浏览: 24
在 Vue 3 中,正确地封装和管理 HTTP 接口通常会使用 Axios 或者 Vue 自带的 Fetch API。以下是使用 Axios 进行封装的一个简单示例:
首先,安装 Axios:
```bash
npm install axios
# 或者
yarn add axios
```
然后,在你的 Vue 组件之外创建一个单独的 `apiService.js` 或类似文件,用于集中管理请求:
```javascript
import axios from 'axios';
const service = axios.create({
baseURL: process.env.VUE_APP_API_BASE_URL, // 根据你的项目配置API基础地址
timeout: 5000, // 超时时间
headers: {
'Content-Type': 'application/json',
Authorization: 'Bearer ' + localStorage.getItem('token'), // 如果需要验证 token
},
});
export default {
get: async (url) => {
try {
const response = await service.get(url);
return response.data;
} catch (error) {
console.error(error);
throw new Error('Failed to fetch data');
}
},
post: async (url, data) => {
try {
const response = await service.post(url, data);
return response.data;
} catch (error) {
console.error(error);
throw new Error('Failed to send data');
}
},
// 其他HTTP方法,如 put、delete 等...
};
```
接着,在需要使用这个服务的地方引入并调用它:
```javascript
import { get, post } from '@/services/apiService';
export default {
async fetchData() {
try {
const data = await get('/your-endpoint');
// 对数据进行处理...
} catch (error) {
console.error(error);
}
},
async sendData(data) {
try {
await post('/your-endpoint', data);
// 数据发送成功后的操作...
} catch (error) {
console.error(error);
}
},
};
```
阅读全文
相关推荐
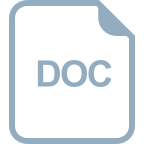
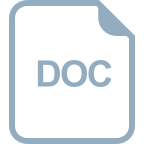
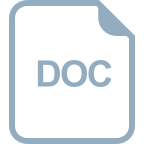

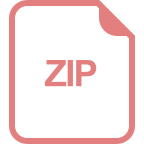
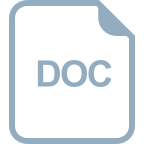
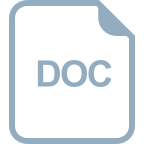
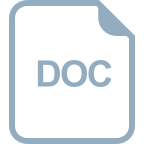
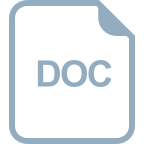
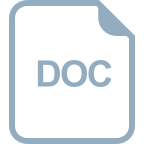
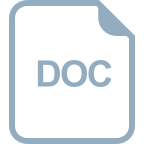







