编程实现输入任意2个小于100的正整数a和b,然后输出100以内能同时整除a和b的所有数(模范例题,至少写成三层),并使用gcc和gdb、Makefile编译和调试
时间: 2024-10-24 14:19:01 浏览: 56
当你需要编写这样的程序时,可以按照以下步骤设计三层结构:
**第一层:主函数入口**
```c
#include <stdio.h>
int main() {
int a, b;
printf("请输入两个小于100的正整数:\n");
scanf("%d %d", &a, &b);
// 如果输入错误,这里可以添加一些边界检查
// 调用函数 find_common_divisors(a, b) 来找出共同因子
int common_factors[] = find_common_divisors(a, b);
if (common_factors) {
print_common_factors(common_factors);
} else {
printf("没有找到100以内的公约数。\n");
}
return 0;
}
```
**第二层:辅助函数 - 查找公共因子**
```c
// 定义find_common_divisors 函数,它接收a和b作为参数,并返回一个包含它们公共因子的数组
int* find_common_divisors(int a, int b) {
int max_num = 100; // 只考虑100以内的数
int gcd = __gcd(a, b); // 使用__gcd函数求最大公约数
int common_factors[max_num / gcd + 1]; // 最大公约数可能是a或b,所以数组大小是100/gcd+1
int index = 0; // 计数器
for (int i = 1; i <= max_num / gcd; i++) {
if (i * gcd <= max_num && common_factors[index] == 0) {
common_factors[index++] = i * gcd; // 将公共因子放入数组
}
}
common_factors[index] = NULL; // 空结束符表示数组结束
return common_factors;
}
// 未在此代码示例中显示的内建函数 __gcd(),用于计算最大公约数
```
**第三层:辅助函数 - 输出公共因子**
```c
void print_common_factors(int* factors) {
if (factors[0]) { // 检查是否有因子存在
printf("100以内能同时整除a和b的数有:\n");
for (int i = 0; factors[i]; i++) {
printf("%d ", factors[i]);
}
printf("\n");
}
}
```
**编译和调试**
为了编译和调试这个程序,你可以创建一个`Makefile`文件,内容如下:
```makefile
CC = gcc
CFLAGS = -Wall -g
all: common_factors
common_factors: common_factors.c
$(CC) $(CFLAGS) -o common_factors common_factors.c
debug: all
gdb -q common_factors
clean:
rm -rf common_factors
```
运行`make`命令构建程序,`make debug`则启动gdb进行调试。
阅读全文
相关推荐


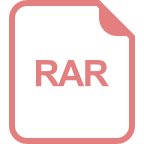
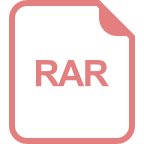









