playfair matlab
时间: 2023-11-11 20:00:59 浏览: 96
Playfair密码是一种古典密码,它使用一个5x5的矩阵来加密明文。在Matlab中,你可以使用以下代码来实现Playfair密码的加密和解密:
```matlab
function ciphertext = playfair_encrypt(plaintext, key)
% Remove spaces and convert to uppercase
plaintext = upper(strrep(plaintext, ' ', ''));
key = upper(strrep(key, ' ', ''));
% Create the Playfair matrix
alphabet = 'ABCDEFGHIKLMNOPQRSTUVWXYZ';
matrix = [key, setdiff(alphabet, key)];
matrix = reshape(matrix, 5, 5);
% Pad plaintext with X if necessary
if mod(length(plaintext), 2) == 1
plaintext(end+1) = 'X';
end
% Encrypt pairs of letters
ciphertext = '';
for i = 1:2:length(plaintext)
pair = plaintext(i:i+1);
row1 = find(matrix == pair(1));
row2 = find(matrix == pair(2));
if row1(1) == row2(1)
% Same row
col1 = mod(row1(2), 5);
col2 = mod(row2(2),5);
if col1 == 0
col1 = 5;
end
if col2 == 0
col2 = 5;
end
ciphertext(end+1:end+2) = [matrix(row1(1), col1), matrix(row2(1), col2)];
elseif row1(2) == row2(2)
% Same column
row1 = mod(row1(1), 5);
row2 = mod(row2(1), 5);
if row1 == 0
row1 = 5;
end
if row2 == 0
row2 = 5;
end
ciphertext(end+1:end+2) = [matrix(row1, row1(2)), matrix(row2, row2(2))];
else
% Rectangle
ciphertext(end+1:end+2) = [matrix(row1(1), row2(2)), matrix(row2(1), row1(2))];
end
end
end
function plaintext = playfair_decrypt(ciphertext, key)
% Remove spaces and convert to uppercase
ciphertext = upper(strrep(ciphertext, ' ', ''));
key = upper(strrep(key, ' ', ''));
% Create the Playfair matrix
alphabet = 'ABCDEFGHIKLMNOPQRSTUVWXYZ';
matrix = [key, setdiff(alphabet, key)];
matrix = reshape(matrix, 5, 5);
% Decrypt pairs of letters
plaintext = '';
for i = 1:2:length(ciphertext)
pair = ciphertext(i:i+1);
row1 = find(matrix == pair(1));
row2 = find(matrix == pair(2));
if row1(1) == row2(1)
% Same row
col1 = mod(row1(2)-2, 5)+1;
col2 = mod(row2(2)-2, 5)+1;
plaintext(end+1:end+2) = [matrix(row1(1), col1), matrix(row2(1), col2)];
elseif row1(2) == row2(2)
% Same column
row1 = mod(row1(1)-2, 5)+1;
row2 = mod(row2(1)-2, 5)+1;
plaintext(end+1:end+2) = [matrix(row1, row1(2)), matrix(row2, row2(2))];
else
% Rectangle
plaintext(end+1:end+2) = [matrix(row1(1), row2(2)), matrix(row2(1), row1(2))];
end
end
end
```
其中,`playfair_encrypt`函数用于加密明文,`playfair_decrypt`函数用于解密密文。你需要传入明文或密文以及密钥作为参数。
阅读全文
相关推荐
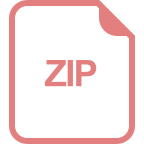
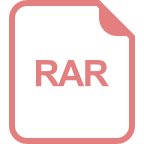
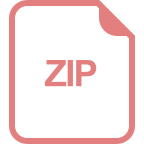
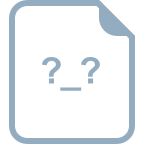
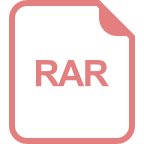
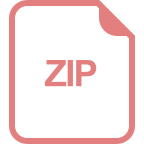
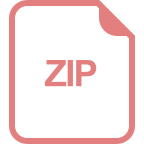
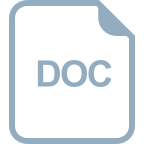
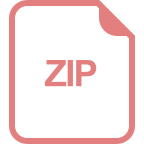
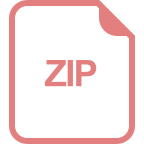
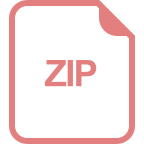
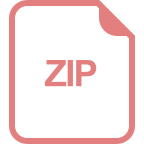
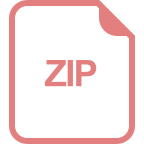
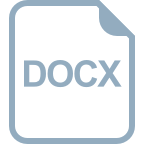