c语言学生管理系统按成绩排序代码
时间: 2024-08-14 21:02:14 浏览: 74
在C语言中,实现一个简单的学生成绩管理系统并按照成绩对记录进行排序可能涉及以下几个步骤。假设我们有一个结构体来存储学生的姓名(name)和成绩(score),我们可以创建一个动态数组或链表来存储这些数据,然后使用qsort函数(标准库中的`<stdlib.h>`)来进行排序。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义学生结构体
typedef struct {
char name[50];
float score;
} Student;
// 比较函数,用于qsort排序
int compare(const void *a, const void *b) {
const Student *sa = (const Student *) a;
const Student *sb = (const Student *) b;
return (sa->score > sb->score) ? 1 : ((sa->score < sb->score) ? -1 : 0);
}
void sortStudents(Student students[], int n) {
qsort(students, n, sizeof(Student), compare);
}
int main() {
// 假设我们有5个学生
Student students[] = {
{"Alice", 85},
{"Bob", 92},
{"Charlie", 78},
{"David", 98},
{"Eve", 80}
};
int num_students = sizeof(students) / sizeof(students);
printf("Before sorting:\n");
for (int i = 0; i < num_students; i++) {
printf("%s: %f\n", students[i].name, students[i].score);
}
// 对学生数组按成绩排序
sortStudents(students, num_students);
printf("\nAfter sorting by score in descending order:\n");
for (int i = 0; i < num_students; i++) {
printf("%s: %f\n", students[i].name, students[i].score);
}
return 0;
}
```
这段代码首先定义了一个学生结构体,然后定义了一个比较函数`compare`来决定升序还是降序排序。`sortStudents`函数接受一个学生数组和其大小作为参数,调用`qsort`函数完成排序。在`main`函数中,我们初始化一个学生数组并展示排序前后的结果。
阅读全文
相关推荐









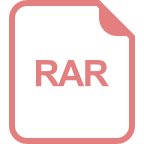








