vue 定时任务调用方法
时间: 2024-08-29 08:00:20 浏览: 16
在Vue.js中,你可以使用`setTimeout`、`setInterval`或`MutationObserver`等JavaScript内置函数来创建定时任务并调用你的自定义方法。这里是一个简单的例子:
```javascript
// 首先,在你的组件内定义一个需要定时调用的方法
data() {
return {
timerId: null,
}
},
methods: {
callMyMethod() {
// 这里是你实际想要执行的方法
console.log('This is my method.');
},
}
// 然后,你可以设置一个定时任务,比如每5秒调用一次
created() {
this.timerId = setInterval(() => {
this.callMyMethod();
}, 5000); // 5000毫秒等于5秒
},
// 当组件销毁时,记得清除定时任务以避免内存泄漏
beforeDestroy() {
clearInterval(this.timerId);
this.timerId = null;
}
```
在上面的例子中,`created`生命周期钩子会在实例创建后立即执行,并开始定时调用`callMyMethod`方法。当组件即将卸载时,使用`beforeDestroy`清理定时器。
相关问题
vue定时任务setInterval
在Vue中使用定时任务setInterval可以在mounted()函数中使用。可以先在methods中定义一个需要执行的方法,然后在mounted()函数中调用该方法,并使用setInterval设置定时器,第一个参数为方法名,不要加括号,第二个参数为时间间隔。例如,在mounted()函数中可以这样写:setInterval(getElevatorList, 1000)。这样就可以每隔1秒钟执行一次getElevatorList方法。
vue quartz定时任务cron表达式
cron表达式是用于定义定时任务调度的一种时间表达式。在Vue中,可以使用vue-quartz插件来实现定时任务的调度。
首先,需要在项目中安装vue-quartz插件。可以通过npm或yarn进行安装:
```
npm install vue-quartz
```
或
```
yarn add vue-quartz
```
安装完成后,在Vue的入口文件main.js中引入并注册vue-quartz插件:
```javascript
import Vue from 'vue';
import VueQuartz from 'vue-quartz';
Vue.use(VueQuartz);
```
接下来,在需要定义定时任务的组件中,使用`<cron>`标签来设置cron表达式:
```vue
<template>
<div>
<h1>定时任务</h1>
<cron v-model="cronExpression" @change="handleCronChange"></cron>
</div>
</template>
<script>
export default {
data() {
return {
cronExpression: ''
};
},
methods: {
handleCronChange(cronExpression) {
// 在这里可以处理cron表达式的变化,并执行相应的操作
console.log('cron表达式变化:', cronExpression);
}
}
};
</script>
```
在上面的示例中,通过`v-model`指令将cron表达式绑定到组件的data属性`cronExpression`上,并通过`@change`事件监听cron表达式的变化。
当用户在页面上选择或输入cron表达式时,`handleCronChange`方法会被调用,并传入新的cron表达式。在该方法中可以根据cron表达式执行相应的定时任务逻辑。
请注意,以上只是一个简单的示例,具体的定时任务逻辑需要根据实际需求进行实现。同时,需要了解cron表达式的语法规则和含义,以确保正确设置定时任务的执行时间。
相关推荐
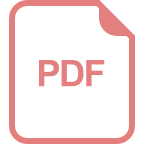
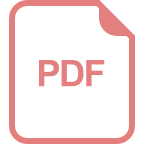
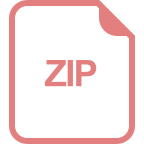












